用C++写一个串口通信的通用代码
时间: 2023-08-31 22:22:46 浏览: 185
### 回答1:
好的,下面是一个使用C语言编写的串口通信的通用代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int set_interface_attribs(int fd, int speed, int parity)
{
struct termios tty;
memset(&tty, 0, sizeof tty);
if (tcgetattr(fd, &tty) != 0) {
printf("error %d from tcgetattr", errno);
return -1;
}
cfsetospeed(&tty, speed);
cfsetispeed(&tty, speed);
tty.c_cflag = (tty.c_cflag & ~CSIZE) | CS8; // 8-bit chars
// disable IGNBRK for mismatched speed tests; otherwise receive break
// as \000 chars
tty.c_iflag &= ~IGNBRK; // disable break processing
tty.c_lflag = 0; // no signaling chars, no echo,
// no canonical processing
tty.c_oflag = 0; // no remapping, no delays
tty.c_cc[VMIN] = 0; // read doesn't block
tty.c_cc[VTIME] = 5; // 0.5 seconds read timeout
tty.c_iflag &= ~(IXON | IXOFF | IXANY); // shut off xon/xoff ctrl
tty.c_cflag |= (CLOCAL | CREAD);// ignore modem controls,
// enable reading
tty.c_cflag &= ~(PARENB | PARODD); // shut off parity
tty.c_cflag |= parity;
tty.c_cflag &= ~CSTOPB;
tty.c_cflag &= ~CRTSCTS;
if (tcsetattr(fd, TCSANOW, &tty) != 0) {
printf("error %d from tcsetattr", errno);
return -1;
}
return 0;
}
void set_blocking(int fd, int should_block)
{
struct termios tty;
memset(&tty, 0, sizeof tty);
if (tcgetattr(fd, &tty) != 0) {
printf("error %d from tggetattr", errno);
return;
}
tty.c_cc[VMIN] = should_block ? 1 : 0;
tty.c_cc[VTIME] = 5; // 0.5 seconds read timeout
if (tcsetattr(fd, TCSANOW, &tty) != 0) {
printf("error %d setting term attributes", errno);
}
}
int main(int argc, char** argv)
{
if (argc != 2) {
printf("Usage: serialport /dev/ttyUSB0\n");
return 1;
}
char *portname = argv[1];
int fd = open(portname, O_RDWR | O_NOCTTY | O_SYNC);
if (fd < 0) {
printf("error %d opening %s: %s", errno, portname, strerror(errno));
return 1;
}
set_interface_attribs(fd, B9600, 0); // set speed to 9600 bps, 8n1 (no parity)
set_blocking(fd, 0); // set no blocking
char buf[256];
int n;
while (1) {
memset(buf, 0, sizeof buf);
n = read(fd, buf, sizeof buf
### 回答2:
要使用C语言写一个串口通信的通用代码,我们可以使用串口相关的库函数来实现。首先,我们需要引入相应的头文件:<stdio.h>、<fcntl.h>、<termios.h>和<unistd.h>。然后,我们可以定义一个函数来初始化串口,并设置相关的参数。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int serial_init(const char *port, int baudrate) {
int fd = open(port, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("Serial port open failed");
return -1;
}
struct termios tty;
tcgetattr(fd, &tty);
// 设置波特率
cfsetospeed(&tty, baudrate);
cfsetispeed(&tty, baudrate);
// 设置数据位、停止位和校验位
tty.c_cflag &= ~PARENB; // 禁用校验位
tty.c_cflag &= ~CSTOPB; // 设置停止位为1
tty.c_cflag &= ~CSIZE; // 清楚数据位设置
tty.c_cflag |= CS8; // 设置数据位为8
// 设置为原始模式,禁用软件流控制
tty.c_cflag &= ~CRTSCTS;
tty.c_cflag |= CREAD | CLOCAL;
// 设置超时的值
tty.c_cc[VTIME] = 10;
tty.c_cc[VMIN] = 0;
// 应用所有的设置
tcsetattr(fd, TCSANOW, &tty);
// 清空串口缓冲区
tcflush(fd, TCIOFLUSH);
return fd;
}
int main() {
int fd = serial_init("/dev/ttyUSB0", B9600);
if (fd < 0) {
return -1;
}
// 通过fd进行读写数据的操作
close(fd); // 关闭串口
return 0;
}
```
在这个例子中,我们使用了`serial_init`函数来初始化串口,并返回一个文件描述符,用于后续的串口读写操作。在`main`函数中,你可以使用这个文件描述符来进行相关的读写操作,并在最后使用`close`函数关闭串口。当然,在实际使用中,你可能还需要处理一些异常情况和错误处理。
### 回答3:
串口通信是一种常见的硬件通信方式,通过串行传输比特流来进行数据交换。在C语言中,我们可以使用标准库函数来实现串口通信。
以下是一个基本的串口通信代码示例:
```c
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main() {
int fd;
char buffer[256];
// 打开串口设备
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY);
if (fd == -1) {
printf("无法打开串口设备!\n");
return -1;
}
// 配置串口参数
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_oflag &= ~OPOST;
tcsetattr(fd, TCSANOW, &options);
// 读取串口数据
int n;
while (1) {
n = read(fd, buffer, sizeof(buffer));
if (n > 0) {
printf("接收到的数据:%s\n", buffer);
}
usleep(1000);
}
// 关闭串口设备
close(fd);
return 0;
}
```
代码中的串口设备路径`/dev/ttyS0`是一个常见的串口设备路径,具体根据不同的操作系统和串口配置可能会有所不同。此外,还需要根据实际需求进行相关的串口参数配置,例如波特率、数据位、停止位等。读取串口数据的方式可以根据具体需求进行修改,上述示例代码仅供参考。
注意,串口通信属于底层硬件通信,需要理解串口通信的相关知识,并且需要在特定的硬件环境中运行和验证。
阅读全文
相关推荐
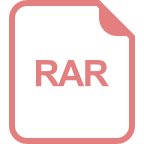
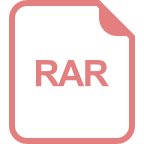
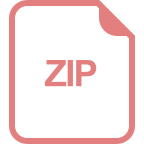















