x_train.shape=(186,19,1) x_train=torch.from_numpy(x_train).type(torch.Tensor)这两句代码如何解释
时间: 2024-06-05 22:07:46 浏览: 152
这两句代码是用来将NumPy数组x_train转换为PyTorch张量的。其中x_train是一个形状为(186,19,1)的NumPy数组,表示有186个样本,每个样本有19个特征,每个特征是一个长度为1的向量。第一行代码中的x_train变量是NumPy数组,第二行代码中的torch.from_numpy(x_train)将该数组转换为PyTorch张量,并使用.type(torch.Tensor)将其转换为浮点型张量。最终,x_train是一个形状为(186,19,1)的PyTorch张量,其中每个元素都是浮点数。
相关问题
import torch import torch.nn as nn import pandas as pd from sklearn.model_selection import train_test_split # 加载数据集 data = pd.read_csv('../dataset/train_10000.csv') # 数据预处理 X = data.drop('target', axis=1).values y = data['target'].values X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) X_train = torch.from_numpy(X_train).float() X_test = torch.from_numpy(X_test).float() y_train = torch.from_numpy(y_train).float() y_test = torch.from_numpy(y_test).float() # 定义LSTM模型 class LSTMModel(nn.Module): def __init__(self, input_size, hidden_size, num_layers, output_size): super(LSTMModel, self).__init__() self.hidden_size = hidden_size self.num_layers = num_layers self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True) self.fc = nn.Linear(hidden_size, output_size) def forward(self, x): h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device) c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device) out, _ = self.lstm(x, (h0, c0)) out = self.fc(out[:, -1, :]) return out # 初始化模型和定义超参数 input_size = X_train.shape[1] hidden_size = 64 num_layers = 2 output_size = 1 model = LSTMModel(input_size, hidden_size, num_layers, output_size) criterion = nn.MSELoss() optimizer = torch.optim.Adam(model.parameters(), lr=0.001) # 训练模型 num_epochs = 100 for epoch in range(num_epochs): model.train() outputs = model(X_train) loss = criterion(outputs, y_train) optimizer.zero_grad() loss.backward() optimizer.step() if (epoch+1) % 10 == 0: print(f'Epoch [{epoch+1}/{num_epochs}], Loss: {loss.item():.4f}') # 在测试集上评估模型 model.eval() with torch.no_grad(): outputs = model(X_test) loss = criterion(outputs, y_test) print(f'Test Loss: {loss.item():.4f}') 我有额外的数据集CSV,请帮我数据集和测试集分离
以下是将数据集和测试集分离的代码示例:
```python
import torch
import torch.nn as nn
import pandas as pd
from sklearn.model_selection import train_test_split
# 加载数据集
data = pd.read_csv('../dataset/train_10000.csv')
# 数据预处理
X = data.drop('target', axis=1).values
y = data['target'].values
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
X_train = torch.from_numpy(X_train).float()
X_test = torch.from_numpy(X_test).float()
y_train = torch.from_numpy(y_train).float()
y_test = torch.from_numpy(y_test).float()
```
以上代码中,我们使用了`sklearn`库的`train_test_split`函数将数据集分割成训练集和测试集。你可以根据需要调整`test_size`参数来确定测试集的比例。然后,我们将数据转换为`torch`张量以供模型使用。
希望这可以帮助到你!如果有任何其他问题,请随时问我。
n_train = train_data.shape[0] train_features = torch.tensor( all_features[:n_train].values,dtype = torch.float32 )
这段代码的作用是将输入的训练数据(train_data)中的特征数据(all_features)转换为 PyTorch 中的张量(tensor)格式,并将它们保存在 train_features 变量中。
具体来说,这段代码首先通过 train_data.shape[0] 获取训练数据中的样本数量(n_train),然后使用 all_features[:n_train].values 获取前 n_train 个样本的特征数据,并将其转换为 numpy 数组格式。接着,使用 torch.tensor 将 numpy 数组转换为 PyTorch 中的张量格式,并指定数据类型为 float32(dtype = torch.float32)。最后,将转换后的张量保存在 train_features 变量中,以便后续使用。
需要注意的是,这段代码假设训练数据(train_data)中的前 n_train 个样本对应的特征数据(all_features)已经被预处理成合适的格式,例如进行了归一化或者标准化处理。如果特征数据还需要进行进一步的预处理,可以在这段代码之前进行处理。
阅读全文
相关推荐
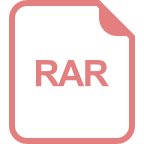
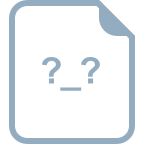














