import numpy as np import pandas as pd import matplotlib as plt from model import MLP import torch.optim as optim import torch import torch.nn as nn import argparse parser = argparse.ArgumentParser() parser.add_argument('--batchSize', type=int, default=4, help='input batch size') parser.add_argument('--nEpochs', type=int, default=100, help='number of epochs to train for') parser.add_argument('--LR', type=float, default=0.001, help='learning rate for net') opt = parser.parse_args() # 数据集预处理 df = pd.read_csv("C:/Users/shen/Desktop/housing.csv", delim_whitespace=True) # Turn into Numpy arrays arr = df.to_numpy(dtype='float') # Split the features and the labels X = arr[:, :-1] y = np.expand_dims(arr[:, -1], 1) ones = np.ones((X.shape[0], 1)) X_new = np.hstack((ones, X)) # Split the dataset into train set and test set indices = np.random.permutation(X_new.shape[0]) train_indices, test_indices = indices[:int(0.9*X_new.shape[0])], indices[int(0.9*X_new.shape[0]):] X_train, X_test = X_new[train_indices, :], X_new[test_indices, :] y_train, y_test = y[train_indices, :], y[test_indices, :] model = MLP() device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu") model.to(device) model.train() optim_model = optim.Adam(model.parameters(), lr=opt.LR)#Adam优化器 # for epoch in range(opt.nEpochs): # # START CODE HERE # # END CODE HERE # torch.save(model.state_dict(),"C:/Users/shen/Desktop/checkpoint/net_weight.pth")帮我解释这里面每行代码的意思
时间: 2023-06-11 11:04:11 浏览: 159
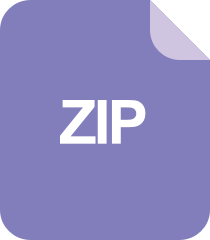
vim-autoimport:Vim插件,用于在不使用LSP的情况下添加导入语句(适用于python等)
这段代码主要是在导入一些Python的库(如numpy,pandas,matplotlib等),以及导入一个叫做MLP的模型。同时还使用了一些PyTorch的库(如optim,nn等)。接下来使用argparse库创建了一个命令行参数解析器,可以通过命令行来指定一些参数(比如batchSize,nEpochs和LR)。最后通过parse_args()函数解析这些参数。
阅读全文
相关推荐
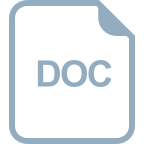
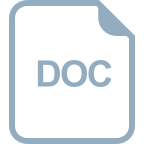















