pytorch自编码器模型使用L1正则化样例
时间: 2023-12-09 20:02:22 浏览: 31
以下是一个使用L1正则化的PyTorch自编码器模型的样例代码:
```python
import torch
import torch.nn as nn
class Autoencoder(nn.Module):
def __init__(self):
super(Autoencoder, self).__init__()
# Encoder layers
self.encoder = nn.Sequential(
nn.Linear(784, 256),
nn.ReLU(),
nn.Linear(256, 64),
nn.ReLU(),
nn.Linear(64, 16)
)
# Decoder layers
self.decoder = nn.Sequential(
nn.Linear(16, 64),
nn.ReLU(),
nn.Linear(64, 256),
nn.ReLU(),
nn.Linear(256, 784),
nn.Sigmoid()
)
# L1 regularization factor
self.reg_factor = 0.001
def forward(self, x):
# Encode input
encoded = self.encoder(x)
# Decode encoded input
decoded = self.decoder(encoded)
return decoded
def loss_function(self, outputs, inputs):
# Compute reconstruction loss
mse_loss = nn.MSELoss()(outputs, inputs)
# Compute L1 regularization loss
l1_loss = 0
for param in self.parameters():
l1_loss += torch.sum(torch.abs(param))
# Combine losses
total_loss = mse_loss + (self.reg_factor * l1_loss)
return total_loss
```
在这个模型中,我们定义了一个包含三个线性层的编码器和一个包含三个线性层的解码器。我们还定义了一个L1正则化系数`reg_factor`,用于控制正则化的强度。
在前向传递过程中,我们首先将输入`x`通过编码器进行编码,并将编码后的结果`encoded`作为解码器的输入,最终得到重构输出`decoded`。
在损失函数中,我们首先计算重构损失(均方误差),然后计算L1正则化损失。最后将两者相加得到总损失。注意,我们只对模型的权重进行L1正则化,而不对偏置进行正则化。
使用此模型进行训练时,只需按照常规方式计算损失并调用`backward()`函数即可。由于L1正则化是通过添加惩罚项来实现的,因此PyTorch会自动计算正则化损失的梯度并将其添加到总梯度中。
相关推荐
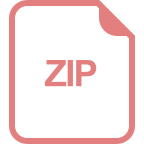
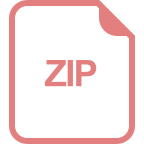
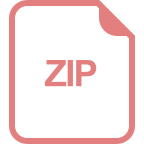
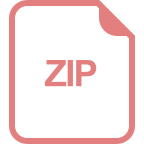
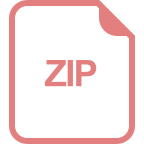
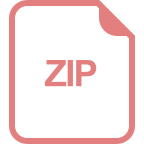