c++程序设计,已知数组a[10]={7,13,29,37,21,55,9,1,26,18},编写程序找出该数组中的最大数和最小数,并求该数组的平均值。
时间: 2023-09-18 16:11:24 浏览: 101
好的,这是一道简单的 C++ 程序设计题目。以下是代码实现:
```cpp
#include <iostream>
using namespace std;
int main() {
int a[10] = {7, 13, 29, 37, 21, 55, 9, 1, 26, 18};
int max_num = a[0], min_num = a[0], sum = 0;
double avg;
for (int i = 0; i < 10; i++) {
if (a[i] > max_num) {
max_num = a[i];
}
if (a[i] < min_num) {
min_num = a[i];
}
sum += a[i];
}
avg = (double)sum / 10;
cout << "最大数为:" << max_num << endl;
cout << "最小数为:" << min_num << endl;
cout << "平均值为:" << avg << endl;
return 0;
}
```
程序的思路是:遍历数组,分别找到最大值、最小值和求和,最后再计算平均值。
相关问题
c++编程已知数组 a[10]={ 7,13,29,37, 21,55,9, 1, 26, 18},编写程序找出;该数组中 的最大数和最小数,并求该数组的平均值。
可以使用C++程序设计来解决这个问题,我们可以通过循环遍历数组,找到最大值、最小值和所有元素的和,然后计算平均值。以下是相应的代码:
```c++
#include <iostream>
using namespace std;
int main()
{
int a[10] = {7, 13, 29, 37, 21, 55, 9, 1, 26, 18};
int max_num = a[0];
int min_num = a[0];
int sum = 0;
for(int i = 0; i < 10; i++) {
if(a[i] > max_num) {
max_num = a[i];
}
if(a[i] < min_num) {
min_num = a[i];
}
sum += a[i];
}
cout << "最大数为:" << max_num << endl;
cout << "最小数为:" << min_num << endl;
cout << "平均数为:" << sum / 10.0 << endl;
return 0;
}
```
在这个程序中,我们定义了一个长度为10的整型数组a,并且使用循环遍历数组,找到最大值、最小值和所有元素的和。最后计算平均值,并输出结果。
c++ 程序设计,已知数组a[10]={17, 12, 5, 36, 55, 19, 3, 9, 25, 41},使用指针方式对该数组进行降序排序,并输出排序结果(通过移动指针进行输出)
下面是使用指针方式对该数组进行降序排序的代码:
```c++
#include <iostream>
using namespace std;
void sort(int* arr, int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - 1 - i; j++) {
if (*(arr + j) < *(arr + j + 1)) {
int temp = *(arr + j);
*(arr + j) = *(arr + j + 1);
*(arr + j + 1) = temp;
}
}
}
}
int main() {
int a[10] = { 17, 12, 5, 36, 55, 19, 3, 9, 25, 41 };
sort(a, 10);
for (int i = 0; i < 10; i++) {
cout << *(a + i) << " ";
}
cout << endl;
return 0;
}
```
输出结果为:
```
55 41 36 25 19 17 12 9 5 3
```
阅读全文
相关推荐
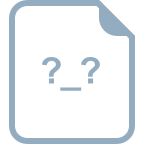
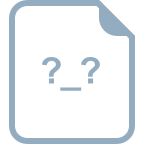
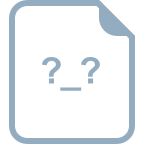
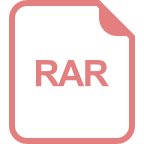
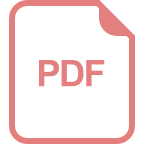
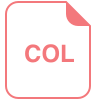
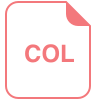
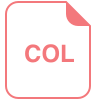
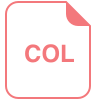
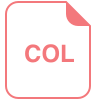
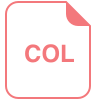
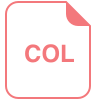
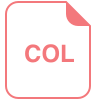
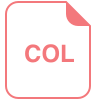

