vue文件上传, Java后台代码
时间: 2024-01-11 10:02:11 浏览: 109
前端Vue文件内的上传代码示例:
```
<template>
<div>
<input type="file" ref="fileInput" @change="uploadFile" />
</div>
</template>
<script>
export default {
methods: {
async uploadFile() {
const file = this.$refs.fileInput.files[0]
const formData = new FormData()
formData.append('file', file)
try {
const response = await this.$http.post('/api/upload', formData)
console.log(response.data)
} catch (error) {
console.log(error)
}
}
}
}
</script>
```
后端Java代码示例:
```
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestPart;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
@RestController
public class UploadController {
private static final String UPLOADED_FOLDER = "/path/to/upload/folder/";
@PostMapping("/api/upload")
public String uploadFile(@RequestPart("file") MultipartFile file) {
if (file.isEmpty()) {
return "Please select a file to upload";
}
try {
// Get the file and save it somewhere
byte[] bytes = file.getBytes();
Path path = Paths.get(UPLOADED_FOLDER + file.getOriginalFilename());
Files.write(path, bytes);
return "You successfully uploaded '" + file.getOriginalFilename() + "'";
} catch (IOException e) {
e.printStackTrace();
return "Failed to upload file";
}
}
}
```
上述示例仅供参考,具体的上传代码需要根据实际需求进行调整。需要注意的是,在使用Java后台处理文件上传时,需要使用MultipartFile类来接收上传的文件。在上传文件之前,需要先设置上传文件的保存路径,并且需要确保该路径是存在的。
阅读全文
相关推荐
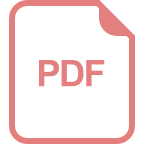
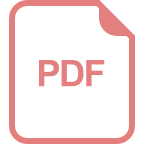
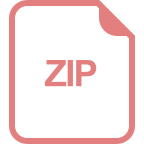
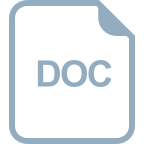
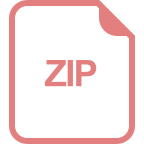
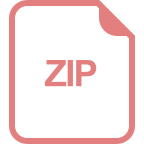
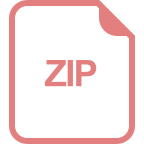
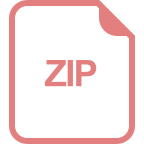
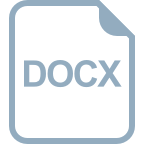
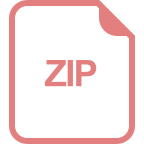
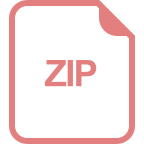
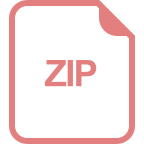
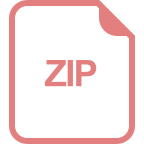
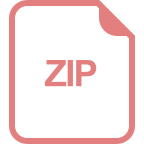



