用python写一个房价爬取代码
时间: 2023-04-08 22:01:59 浏览: 78
好的,我可以回答这个问题。以下是一个简单的Python代码,可以用来爬取房价信息:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com/house-prices'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
prices = soup.find_all('div', class_='price')
for price in prices:
print(price.text)
```
请注意,这只是一个示例代码,实际上,爬取房价信息可能需要更复杂的代码,也需要遵守相关法律法规。
相关问题
请写一段python代码帮我爬取合肥市房价
首先,你需要安装爬虫所需的库,例如 `requests` 和 `BeautifulSoup`。然后,你可以使用以下代码来爬取合肥市房价信息:
```
import requests
from bs4 import BeautifulSoup
def crawl_hefei_house_prices():
# 请求网页
url = "http://www.example.com/hefei/house/prices" # 这里填写你要爬取的网页地址
response = requests.get(url)
html = response.text
# 解析网页
soup = BeautifulSoup(html, 'html.parser')
prices = []
for div in soup.find_all('div', class_='price'): # 寻找所有class属性为price的div元素
price = div.text # 获取div元素的文本内容
prices.append(price)
return prices
prices = crawl_hefei_house_prices()
print(prices)
```
这段代码会访问你所提供的网页,然后使用 `BeautifulSoup` 库解析网页,找到所有 `class` 属性为 `price` 的 `div` 元素,并获取它们的文本内容,最后将获取到的房价信息存储在列表中并返回。
注意:上述代码只是为了演示如何爬取合肥市房价信息,并不能直接使用。你需要根据你要爬取的网页来修改网页地址和解析方式。
帮我写一个能够爬取查询北京潘家园附近租房价的代码
当涉及到爬取网页数据时,我们需要使用第三方库来发送HTTP请求并解析HTML文档。在Python中,常用的库是`requests`和`beautifulsoup4`。下面是一个简单的示例代码,用于爬取并提取北京潘家园附近的租房价信息:
```python
import requests
from bs4 import BeautifulSoup
def crawl_rent_prices():
# 构造URL
url = "https://www.example.com/panjiayuan" # 替换为实际的租房信息网站URL
# 发送HTTP GET请求
response = requests.get(url)
# 解析HTML内容
soup = BeautifulSoup(response.text, "html.parser")
# 提取租房价信息
prices = soup.find_all("span", class_="rent-price") # 根据实际网页结构和CSS类名进行调整
# 打印租房价信息
for price in prices:
print(price.text)
# 调用函数进行爬取并打印结果
crawl_rent_prices()
```
请注意,上述代码仅仅是一个示例,需要您根据实际的租房信息网站的结构进行调整。您需要替换`url`变量为您要爬取的网站的URL,并根据实际网页结构和CSS类名来提取租房价信息。
另外,请务必确保您在爬取网站数据时遵守相关的法律法规,并尊重网站的使用规则和隐私政策。
相关推荐
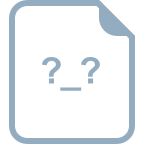












