使用Python把多个子文件夹下的excel合并为一个excel
时间: 2024-01-26 11:04:23 浏览: 49
可以使用Python中的pandas库来实现这个功能。以下是一个代码示例:
```python
import pandas as pd
import os
# 设置工作目录
os.chdir('path/to/parent/folder')
# 获取所有的excel文件名
excel_names = []
for root, dirs, files in os.walk('.'):
for file in files:
if file.endswith('.xlsx'):
excel_names.append(os.path.join(root, file))
# 将所有的excel文件合并为一个DataFrame
df = pd.DataFrame()
for name in excel_names:
temp_df = pd.read_excel(name)
df = pd.concat([df, temp_df], ignore_index=True)
# 将DataFrame写入一个excel文件中
with pd.ExcelWriter('merged.xlsx') as writer:
df.to_excel(writer, index=False)
```
这个代码会遍历指定的父文件夹下的所有子文件夹,获取所有以`.xlsx`结尾的excel文件名,并将它们合并为一个DataFrame。最后,将这个DataFrame写入一个新的excel文件中。
相关问题
使用Python将某个文件夹下的多个Excel文件合并到一个文件中
可以使用Python中的pandas库来实现将多个Excel文件合并到一个文件中。
首先需要安装pandas库,可以使用以下命令进行安装:
```
pip install pandas
```
然后,可以使用以下代码实现将某个文件夹下的多个Excel文件合并到一个文件中:
```python
import os
import pandas as pd
# 文件夹路径
folder_path = 'path/to/folder'
# 获取文件夹下所有Excel文件名
file_names = [name for name in os.listdir(folder_path) if name.endswith('.xlsx')]
# 创建一个空的DataFrame
merged_df = pd.DataFrame()
# 遍历所有Excel文件,将数据合并到merged_df中
for file_name in file_names:
file_path = os.path.join(folder_path, file_name)
df = pd.read_excel(file_path)
merged_df = pd.concat([merged_df, df], ignore_index=True)
# 将合并后的数据保存到一个新的Excel文件中
merged_df.to_excel('merged.xlsx', index=False)
```
其中,需要将`folder_path`修改为需要合并Excel文件的文件夹路径,然后运行代码即可。最终合并后的数据将保存到一个名为`merged.xlsx`的Excel文件中。
python合并文件夹下多个excel表格
下面是一个Python程序示例,可以将文件夹下的多个Excel表格合并成一个文件:
```python
import os
import pandas as pd
# 定义要合并的文件夹路径
folder_path = r"C:\data"
# 获取文件夹下所有的Excel文件
file_names = [f for f in os.listdir(folder_path) if f.endswith('.xlsx')]
# 定义空的DataFrame用于存储所有数据
all_data = pd.DataFrame()
# 循环遍历每个Excel文件,将数据读取到all_data中
for file_name in file_names:
file_path = os.path.join(folder_path, file_name)
data = pd.read_excel(file_path)
all_data = pd.concat([all_data, data], ignore_index=True)
# 将所有数据保存到一个新的Excel文件中
output_file = os.path.join(folder_path, 'merged_data.xlsx')
all_data.to_excel(output_file, index=False)
```
在这个示例中,我们使用了`os`和`pandas`两个Python库。`os`库用于处理文件和文件夹,`pandas`库用于读取和处理Excel数据。首先我们定义了要合并的文件夹路径,然后使用`os.listdir`函数获取文件夹下的所有文件名。接下来,我们循环遍历每个Excel文件,使用`pd.read_excel`函数将数据读取到一个DataFrame中,然后使用`pd.concat`函数将所有数据合并到一起。最后,我们使用`pd.to_excel`函数将所有数据保存到一个新的Excel文件中。
相关推荐
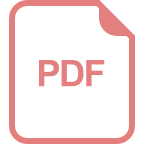
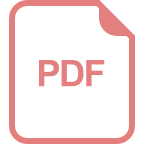
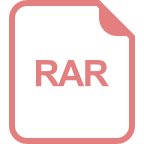












