试编写一个函数,在数组R(已正序排列)中进行折半查找某个值k,找到则返回其位置,否则返回0。
时间: 2024-02-11 12:08:55 浏览: 38
好的,以下是一个在已经正序排列的数组中进行折半查找的函数的实现:
```c
int binary_search(int R[], int n, int k) {
int left = 0, right = n - 1, mid;
while (left <= right) {
mid = (left + right) / 2;
if (R[mid] == k) {
return mid + 1; // 找到了,返回位置(从1开始计数)
} else if (R[mid] < k) {
left = mid + 1; // 在右半部分查找
} else {
right = mid - 1; // 在左半部分查找
}
}
return 0; // 没有找到
}
```
函数的参数是一个已经正序排列的数组R、数组的长度n和要查找的值k。函数会使用折半查找的方法,在数组R中查找k。如果找到了k,函数会返回它在数组中的位置(从1开始计数),否则返回0。需要注意的是,这个函数的时间复杂度为O(log n),比线性查找的时间复杂度O(n)更加高效。
相关问题
vc++写一个折半查找方法要能输入任何一个数,然后返回这个数在排序后的数组中的位置。
### 回答1:
这是一个折半查找的方法的示例,它接受一个数字作为输入,并返回该数字在排序后的数组中的位置:
```
int binary_search(int num, int* array, int array_size) {
int left = 0;
int right = array_size - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (array[mid] == num) {
return mid;
}
if (array[mid] < num) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
```
在这个方法中,我们使用了一个循环来进行折半查找。我们设置两个指针 `left` 和 `right`,它们分别指向数组的左端和右端。然后我们计算中间位置的索引,如果数组中间位置的值等于要查找的数字,那么我们就返回这个索引。如果数组中间位置的值小于要查找的数字,那么我们就更新 `left` 指针的值,使它指向中间位置的右边。如果数组中间位置的值大于要查找的数字,那么我们就更新 `right` 指针的值,使它指向中间位置的左边。这样,我们就可以通过不断缩小查找范围来找到要查找的数字的位置。
### 回答2:
折半查找(Binary Search)是一种高效的查找算法,可以在已排序的数组中查找指定元素,并返回其在数组中的位置。
首先,我们需要定义一个函数来实现折半查找。该函数接受三个参数:数组arr、要查找的数num以及数组的范围left和right。其中,left表示查找范围的左边界,right表示查找范围的右边界。
步骤如下:
1. 判断left是否大于right,若为真则表示查找范围为空,返回-1(表示未找到);
2. 计算中间位置mid = (left + right) / 2;
3. 判断num是否等于数组中的第mid个元素arr[mid],若为真则返回mid(表示找到了);
4. 判断num是否小于arr[mid],若为真则递归调用步骤1中的函数,传入参数arr、num、left和mid-1,返回值即为目标数在排序后的数组中的位置;
5. 若上述判断都不满足,则递归调用步骤1中的函数,传入参数arr、num、mid+1和right,返回值即为目标数在排序后的数组中的位置。
下面是实现该函数的代码:
def binarySearch(arr, num, left, right):
if left > right:
return -1
mid = (left + right) // 2
if num == arr[mid]:
return mid
if num < arr[mid]:
return binarySearch(arr, num, left, mid-1)
return binarySearch(arr, num, mid+1, right)
接下来,我们可以编写一个主程序来使用该函数。首先,输入一个待查找的数num和已排序的数组arr。然后,调用binarySearch函数,并传入参数arr、num、0和数组长度减1。最后,根据函数返回的结果判断结果,若返回-1则表示未找到,否则表示找到,并输出结果。
num = int(input("请输入一个数:"))
arr = [3, 7, 10, 15, 18, 20, 23, 25, 30] # 假设数组已经排序
position = binarySearch(arr, num, 0, len(arr)-1)
if position == -1:
print("未找到该数")
else:
print("该数在排序后的数组中的位置为:", position)
通过以上步骤,我们可以输入任何一个数,并返回该数在排序后的数组中的位置。
### 回答3:
折半查找(Binary Search)是一种用于在有序数组中查找指定元素的算法。以下是一个简单的使用C语言编写的折半查找方法,可以输入任意一个数,并返回该数在排序后的数组中的位置。
```c
#include<stdio.h>
int binarySearch(int arr[], int n, int key){
int left = 0;
int right = n - 1;
while(left <= right){
int mid = (left + right) / 2;
if(arr[mid] == key){
return mid;
}
else if(arr[mid] < key){
left = mid + 1;
}
else{
right = mid - 1;
}
}
return -1; // 如果未找到元素,则返回-1
}
int main(){
int n; // 数组长度
int arr[100]; // 声明一个数组
int key; // 要查找的元素
// 输入数组长度
printf("请输入数组长度:");
scanf("%d", &n);
// 输入数组元素
printf("请输入有序数组元素:\n");
for(int i=0; i<n; i++){
scanf("%d", &arr[i]);
}
// 输入要查找的元素
printf("请输入要查找的元素:");
scanf("%d", &key);
// 调用折半查找方法
int position = binarySearch(arr, n, key);
// 输出结果
if(position != -1){
printf("%d 在排序后的数组中的位置是%d。\n", key, position);
}
else{
printf("%d 未找到在排序后的数组中。\n", key);
}
return 0;
}
```
上述代码首先输入数组的长度,然后输入有序数组的元素,再输入要查找的元素。接着调用折半查找方法,如果找到了该元素,则输出它在排序后的数组中的位置,否则输出未找到的信息。
C语言编程采用折半查找法在10个有序数中寻找某个数,如果找到, 输出其所在数组中下标的位置,否则,输出没找到。
好的,这是一个经典的算法问题。以下是C语言程序实现折半查找法:
```c
#include <stdio.h>
int binarySearch(int arr[], int n, int target)
{
int left = 0;
int right = n - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] > target) {
right = mid - 1;
} else {
left = mid + 1;
}
}
return -1;
}
int main()
{
int arr[10] = {2, 4, 6, 8, 10, 12, 14, 16, 18, 20};
int target = 12;
int index = binarySearch(arr, 10, target);
if (index == -1) {
printf("没找到\n");
} else {
printf("找到了,下标为%d\n", index);
}
return 0;
}
```
在这个例子中,我们定义了一个名为binarySearch的函数,它接受一个有序数组、数组的大小和目标值作为参数,并返回目标值在数组中的下标位置(如果目标值存在于数组中),否则返回-1。在主函数中,我们定义了一个有序数组,然后调用binarySearch函数找到目标值的位置。如果找到了,我们输出它的下标位置,否则输出“没找到”。
相关推荐
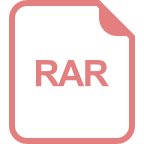












