实现一个照片采集的功能,要求前后端都要有,照片压缩200k,具体代码如何实现,用若依框架实现
时间: 2024-02-17 13:02:05 浏览: 22
好的,针对您的问题,我将向您介绍如何使用若依框架实现一个照片采集的功能,包括前后端实现和照片压缩的功能。
前端实现:
1. 在页面中添加一个上传照片的按钮,并绑定一个事件处理函数。
```
<el-button type="primary" @click="uploadPhoto">上传照片</el-button>
```
2. 在事件处理函数中,调用ElementUI的上传组件,并设置上传的参数和上传成功后的回调函数。
```
uploadPhoto() {
this.$refs.uploadPhoto.uploadFiles(this.fileList)
},
uploadUrl: '/api/photo/upload',
headers: {
'Authorization': 'Bearer ' + getToken()
},
onSuccess(response, file, fileList) {
this.fileList = fileList
this.$message.success('上传成功')
},
onError() {
this.$message.error('上传失败')
},
```
其中,uploadUrl是上传接口的地址,headers是请求头部,onSuccess是上传成功后的回调函数,onError是上传失败后的回调函数。
3. 在回调函数中,将上传成功后的图片地址保存到服务器。
```
@PostMapping("/api/photo/upload")
public AjaxResult uploadPhoto(MultipartFile file) throws Exception {
String fileName = file.getOriginalFilename();
String filePath = RuoYiConfig.getProfile() + "/photo/" + fileName;
String url = "/profile/photo/" + fileName;
File dest = new File(filePath);
FileUtils.copyInputStreamToFile(file.getInputStream(), dest);
return AjaxResult.success("上传成功", url);
}
```
后端实现:
1. 首先,需要在Spring Boot中添加文件上传的依赖项。
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
```
2. 然后,编写一个上传文件的接口。
```
@PostMapping("/api/photo/upload")
public AjaxResult uploadPhoto(MultipartFile file) throws Exception {
String fileName = file.getOriginalFilename();
String filePath = RuoYiConfig.getProfile() + "/photo/" + fileName;
String url = "/profile/photo/" + fileName;
File dest = new File(filePath);
FileUtils.copyInputStreamToFile(file.getInputStream(), dest);
return AjaxResult.success("上传成功", url);
}
```
其中,RuoYiConfig.getProfile()是获取系统的存储目录,file.getOriginalFilename()是获取上传文件的原始文件名,FileUtils.copyInputStreamToFile()是将上传的文件保存到系统中。
照片压缩实现:
1. 在前端上传照片的回调函数中,添加照片压缩的代码。
```
onSuccess(response, file, fileList) {
this.fileList = fileList
this.compressPhoto(file.raw, 200 * 1024, (result) => {
this.uploadPhoto(result)
})
this.$message.success('上传成功')
},
```
其中,compressPhoto是照片压缩的函数,file.raw是上传的原始文件,200 * 1024是指定的压缩后的文件大小,result是压缩后的文件。
2. 编写照片压缩的函数。
```
compressPhoto(file, maxSize, callback) {
let reader = new FileReader()
reader.readAsDataURL(file)
reader.onload = (e) => {
let img = new Image()
img.src = e.target.result
img.onload = () => {
let canvas = document.createElement('canvas')
let context = canvas.getContext('2d')
let originWidth = img.width
let originHeight = img.height
let maxWidth = 800, maxHeight = 800
let targetWidth = originWidth, targetHeight = originHeight
if (originWidth > maxWidth || originHeight > maxHeight) {
if (originWidth / originHeight > maxWidth / maxHeight) {
targetWidth = maxWidth
targetHeight = Math.round(maxWidth * (originHeight / originWidth))
} else {
targetHeight = maxHeight
targetWidth = Math.round(maxHeight * (originWidth / originHeight))
}
}
canvas.width = targetWidth
canvas.height = targetHeight
context.clearRect(0, 0, targetWidth, targetHeight)
context.drawImage(img, 0, 0, targetWidth, targetHeight)
canvas.toBlob((blob) => {
if (blob.size > maxSize) {
this.compressPhoto(blob, maxSize, callback)
} else {
callback(blob)
}
}, 'image/jpeg', 0.9)
}
}
},
```
其中,maxSize是指定的压缩后的文件大小,callback是压缩后的文件回调函数,canvas.toBlob()是将压缩后的文件转换成Blob对象,可以直接上传到服务器。
以上就是使用若依框架实现照片采集的具体代码实现过程,希望对您有所帮助。
相关推荐
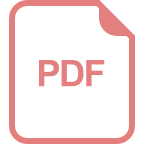
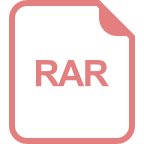
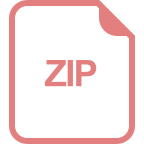














