在VS2019用C++写一个满足多态和继承的例子
时间: 2024-02-17 19:00:57 浏览: 34
好的,下面是一个简单的例子:
```cpp
#include <iostream>
using namespace std;
// 基类 Animal
class Animal {
public:
virtual void speak() {
cout << "动物发出声音" << endl;
}
};
// 派生类 Dog,继承自 Animal
class Dog : public Animal {
public:
void speak() {
cout << "汪汪汪" << endl;
}
};
// 派生类 Cat,继承自 Animal
class Cat : public Animal {
public:
void speak() {
cout << "喵喵喵" << endl;
}
};
// 派生类 Sheep,继承自 Animal
class Sheep : public Animal {
public:
void speak() {
cout << "咩咩咩" << endl;
}
};
int main() {
Animal *animal;
Dog dog;
Cat cat;
Sheep sheep;
animal = &dog;
animal->speak();
animal = &cat;
animal->speak();
animal = &sheep;
animal->speak();
return 0;
}
```
在这个例子中,Animal 是一个基类,而 Dog、Cat、Sheep 都是继承自 Animal 的派生类。基类中有一个虚函数 speak(),在派生类中都重写了这个函数,实现了多态。在 main() 函数中,我们通过指向基类对象的指针来调用不同的派生类中的 speak() 函数,实现了多态和继承。
相关推荐
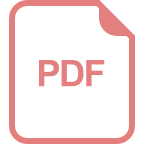














