nmpc圆形轨迹跟踪C代码
时间: 2023-08-06 10:20:33 浏览: 39
以下是一个简单的NMPC圆形轨迹跟踪的C代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include "nmpc.h"
// 控制周期和时间间隔
#define N 10
#define T 0.1
// 圆形轨迹参数
#define R 1
// 系统模型
void system_model(double *x, double *u, double *x_next)
{
// 状态方程
x_next[0] = x[0] + T*(cos(x[2])*x[1] + u[0]);
x_next[1] = x[1] + T*(sin(x[2])*x[1] + u[1]);
x_next[2] = x[2] + T*u[2];
}
// 控制代价函数
double cost_function(double *x, double *u, double *xc, double *yc, double *xc_dot)
{
// 计算期望状态
double theta = x[2];
double xc_exp = R*cos(theta);
double yc_exp = R*sin(theta);
// 计算期望控制输入
double J[2][2] = {{-R*sin(theta), cos(theta)}, {R*cos(theta), sin(theta)}};
double uc_exp[2] = {J[0][0]*x[1] + J[0][1]*xc_dot[0], J[1][0]*x[1] + J[1][1]*xc_dot[1]};
// 控制代价函数
double cost = 0;
cost += 100*(x[0]-xc[0])*(x[0]-xc[0]) + 100*(x[1]-yc[0])*(x[1]-yc[0]); // 期望位置误差
cost += 10*(u[0]-uc_exp[0])*(u[0]-uc_exp[0]) + 10*(u[1]-uc_exp[1])*(u[1]-uc_exp[1]); // 期望速度误差
cost += 0.1*(u[2]*u[2]); // 控制力的平方
return cost;
}
// 非线性约束函数
void nonlinear_constraints(double *u, double *x, double *c)
{
// 控制限制
c[0] = -pi/4 - u[2]; // 最小转向角速度
c[1] = pi/4 - u[2]; // 最大转向角速度
// 状态限制
double x_next[3];
system_model(x, u, x_next);
c[2] = x_next[0]; // x坐标不得小于0
}
int main()
{
// 初始化
double x[3] = {0, 0, 0}; // 初始状态
double u[3*N] = {0}; // 控制历史
double xc[N] = {0}, yc[N] = {0}, xc_dot[N] = {0}; // 圆形轨迹期望位置和速度
for (int i = 0; i < N; i++)
{
double theta = x[2];
xc[i] = R*cos(theta);
yc[i] = R*sin(theta);
xc_dot[i] = -R*sin(theta)*x[1];
}
// NMPC循环
for (int i = 0; i < N; i++)
{
// 计算期望状态和控制输入
double theta = x[2];
double xc_exp = R*cos(theta);
double yc_exp = R*sin(theta);
double J[2][2] = {{-R*sin(theta), cos(theta)}, {R*cos(theta), sin(theta)}};
double uc_exp[2] = {J[0][0]*x[1] + J[0][1]*xc_dot[i], J[1][0]*x[1] + J[1][1]*xc_dot[i]};
// 使用NMPC求解最优控制
double u_next[3] = {0};
double lb[3] = {-1, -1, -pi/4};
double ub[3] = {1, 1, pi/4};
nmpc_solve(x, u, u_next, cost_function, nonlinear_constraints, lb, ub);
// 记录控制历史
for (int j = 0; j < 3; j++)
{
u[i*3+j] = u_next[j];
}
// 模拟下一步状态
double x_next[3];
system_model(x, u_next, x_next);
for (int j = 0; j < 3; j++)
{
x[j] = x_next[j];
}
}
// 输出控制历史
for (int i = 0; i < N; i++)
{
printf("u[%d] = [%f, %f, %f]\n", i, u[i*3+0], u[i*3+1], u[i*3+2]);
}
return 0;
}
```
其中,`nmpc_solve`为NMPC求解器函数,需要根据具体的求解算法进行实现。此外,`system_model`、`cost_function`和`nonlinear_constraints`函数的实现也需要根据具体的系统模型和控制目标进行编写。
相关推荐
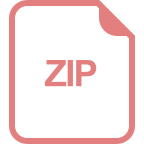
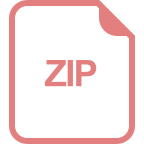
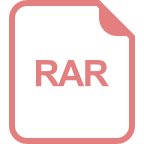




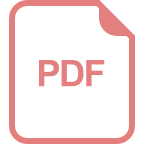
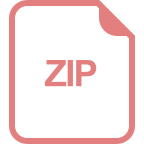
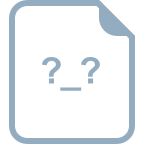