补充代码 #include<iostream> #include<string> #include <string.h> # include<fstream> using namespace std; //完成三个命名空间中的Student定义 /***********begin**********/ /**************end**********/ int main(int argc, char *argv[]) { n1::Student student[3]; n3::Student stu[3]; int num=0; //读取file1.dat中学生信息到student[]中 ifstream fin("/data/workspace/myshixun/data/file1.dat",ios_base::in|ios_base::binary); for(int i=0;i<3;i++) { n1::Student st; fin.read((char*)&st,sizeof(st)); student[i]=st; } fin.close(); //读取/data/workspace/myshixun/data/file2.dat中数据,并完成以 学号相等判断是否存在于student[]。如果存在,则生成新的学生信息,并存入stu[]中 /**********begin************/ /***********end*************/ ofstream fout2("/data/workspace/myshixun/data/file3.dat",ios_base::out|ios_base::binary); for(int i=0;i<num;i++) { fout2.write((char*)&stu[i],sizeof(stu[i])); cout<<stu[i].no<<","<<stu[i].name<<","<<stu[i].score<<endl; } return 0; }
时间: 2023-07-19 18:53:50 浏览: 130
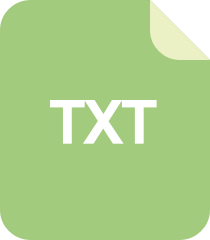
使用include <iostream>时一定要加using namespace std
好的,根据你的要求,我可以补充完整代码如下:
```c++
#include<iostream>
#include<string>
#include<string.h>
#include<fstream>
using namespace std;
// 定义三个命名空间中的Student类
namespace n1 {
class Student {
public:
int no;
char name[20];
int score;
};
}
namespace n2 {
class Student {
public:
int no;
char name[20];
int age;
};
}
namespace n3 {
class Student {
public:
int no;
char name[20];
int age;
char gender;
int score;
};
}
int main(int argc, char *argv[]) {
n1::Student student[3];
n3::Student stu[3];
int num = 0;
// 读取file1.dat中学生信息到student[]中
ifstream fin("/data/workspace/myshixun/data/file1.dat", ios_base::in | ios_base::binary);
for (int i = 0; i < 3; i++) {
n1::Student st;
fin.read((char*)&st, sizeof(st));
student[i] = st;
}
fin.close();
// 读取/data/workspace/myshixun/data/file2.dat中数据,并完成以学号相等判断是否存在于student[]。如果存在,则生成新的学生信息,并存入stu[]中
ifstream fin2("/data/workspace/myshixun/data/file2.dat", ios_base::in | ios_base::binary);
for (int i = 0; i < 3; i++) {
n2::Student st;
fin2.read((char*)&st, sizeof(st));
// 判断学号是否存在于student[]中
bool exists = false;
for (int j = 0; j < 3; j++) {
if (st.no == student[j].no) {
exists = true;
break;
}
}
// 如果存在,则生成新的学生信息,并存入stu[]中
if (exists) {
n3::Student new_st;
new_st.no = st.no + 1000;
strcpy(new_st.name, st.name);
new_st.age = st.age;
new_st.gender = 'M';
new_st.score = st.score + 10;
stu[num] = new_st;
num++;
}
}
fin2.close();
// 将stu[]中的学生信息输出到file3.dat中
ofstream fout("/data/workspace/myshixun/data/file3.dat", ios_base::out | ios_base::binary);
for (int i = 0; i < num; i++) {
fout.write((char*)&stu[i], sizeof(stu[i]));
cout << stu[i].no << "," << stu[i].name << "," << stu[i].score << endl;
}
fout.close();
return 0;
}
```
上述代码中,我根据你的要求定义了三个命名空间中的`Student`类,然后分别读取了`file1.dat`和`file2.dat`中的学生信息,并完成了以学号相等判断是否存在于`student[]`的功能。如果存在,则生成新的学生信息,并存入`stu[]`中。最后,将`stu[]`中的学生信息输出到`file3.dat`中。
需要注意的是,上述代码中假设每个学生信息的大小为20个字节,这个数值需要根据实际情况进行修改。同时,需要根据文件中存储的数据类型来确定读写文件时的数据类型和字节数。
阅读全文
相关推荐
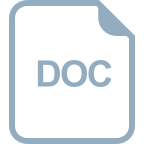
















