线性探测法 hash c++代码
时间: 2023-12-08 22:05:02 浏览: 81
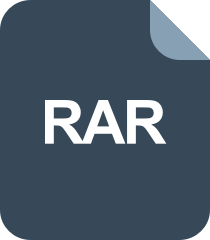
hash算法演示C++

下面是一个简单的线性探测法哈希表的实现(C语言):
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 1000
struct hash_node {
char *key;
char *value;
};
struct hash_table {
struct hash_node *table[TABLE_SIZE];
};
unsigned int hash_function(const char *key) {
unsigned int hash = 0;
int i;
for (i = 0; i < strlen(key); i++) {
hash += key[i];
hash += (hash << 10);
hash ^= (hash >> 6);
}
hash += (hash << 3);
hash ^= (hash >> 11);
hash += (hash << 15);
return hash % TABLE_SIZE;
}
struct hash_table *hash_create() {
struct hash_table *hash = malloc(sizeof(struct hash_table));
int i;
for (i = 0; i < TABLE_SIZE; i++) {
hash->table[i] = NULL;
}
return hash;
}
void hash_destroy(struct hash_table *hash) {
int i;
for (i = 0; i < TABLE_SIZE; i++) {
struct hash_node *node = hash->table[i];
if (node != NULL) {
free(node->key);
free(node->value);
free(node);
}
}
free(hash);
}
void hash_set(struct hash_table *hash, const char *key, const char *value) {
unsigned int slot = hash_function(key);
struct hash_node *node = hash->table[slot];
int i = 0;
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
free(node->value);
node->value = strdup(value);
return;
}
i++;
slot = (slot + i) % TABLE_SIZE;
node = hash->table[slot];
}
node = malloc(sizeof(struct hash_node));
node->key = strdup(key);
node->value = strdup(value);
hash->table[slot] = node;
}
char *hash_get(struct hash_table *hash, const char *key) {
unsigned int slot = hash_function(key);
struct hash_node *node = hash->table[slot];
int i = 0;
while (node != NULL) {
if (strcmp(node->key, key) == 0) {
return node->value;
}
i++;
slot = (slot + i) % TABLE_SIZE;
node = hash->table[slot];
}
return NULL;
}
int main() {
struct hash_table *hash = hash_create();
hash_set(hash, "name", "John");
hash_set(hash, "age", "30");
printf("Name: %s, Age: %s\n", hash_get(hash, "name"), hash_get(hash, "age"));
hash_destroy(hash);
return 0;
}
```
这个哈希表使用了一个简单的哈希函数,可以根据需要进行修改。在这个哈希表中,冲突的时候使用了线性探测法来解决。
阅读全文
相关推荐




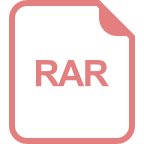
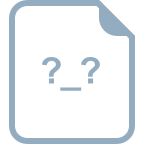
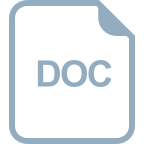









