用python实现利用感知器进行二分类
时间: 2023-09-20 17:05:03 浏览: 75
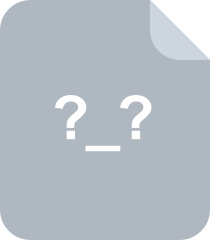
04感知器实现二分类任务.py
感知器是一种二分类的线性分类算法,可以用Python实现。以下是一个简单的感知器实现示例:
```python
import numpy as np
class Perceptron:
def __init__(self, learning_rate=0.1, epochs=50):
self.learning_rate = learning_rate
self.epochs = epochs
def fit(self, X, y):
n_samples, n_features = X.shape
# Initialize weights and bias
self.weights = np.zeros(n_features)
self.bias = 0
# Training loop
for epoch in range(self.epochs):
for i in range(n_samples):
# Activation function
activation = np.dot(X[i], self.weights) + self.bias
# Prediction
y_pred = np.where(activation >= 0, 1, 0)
# Weight and bias update
self.weights += self.learning_rate * (y[i] - y_pred) * X[i]
self.bias += self.learning_rate * (y[i] - y_pred)
def predict(self, X):
# Activation function
activation = np.dot(X, self.weights) + self.bias
# Prediction
y_pred = np.where(activation >= 0, 1, 0)
return y_pred
```
其中,`fit`方法用于训练感知器,`predict`方法用于进行预测。使用示例:
```python
# Sample data
X = np.array([[2, 1], [3, 4], [4, 2], [3, 1]])
y = np.array([0, 1, 1, 0])
# Initialize and train perceptron
perceptron = Perceptron()
perceptron.fit(X, y)
# Predict
X_test = np.array([[1, 1], [4, 3]])
y_pred = perceptron.predict(X_test)
print(y_pred) # [0 1]
```
在这个例子中,我们使用一个简单的样本数据进行训练和预测。感知器的训练过程是通过迭代更新权重和偏差完成的,每个样本点的预测值与真实值的差异会影响权重和偏差的更新。最终,感知器会得到一个可以将样本数据分为两类的决策边界。
阅读全文
相关推荐
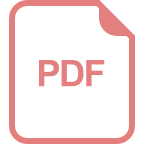
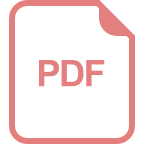
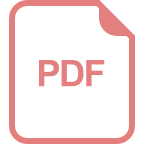


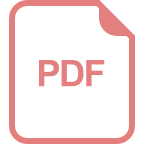
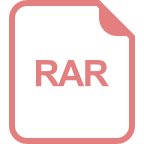
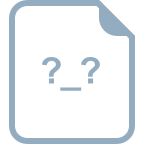
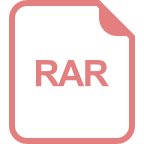
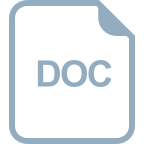
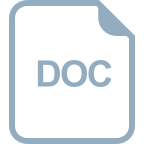
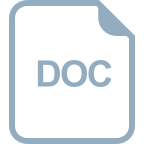
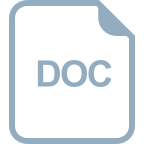
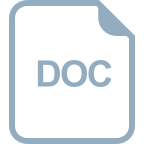
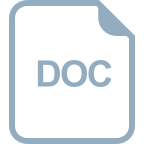

