用java写一个统计单词的程序
时间: 2023-11-16 22:06:25 浏览: 156
好的,您可以使用Java中的HashTable或者Map来实现统计单词的程序。首先,您需要读取文本文件并将其中的字符串分割成单词。然后创建一个HashMap来存储每个单词及其出现的次数。最后,遍历完整个文本文件后,输出HashMap中所有单词及其出现次数即可。以下是示例代码:
```
import java.io.*;
import java.util.*;
public class WordCount {
public static void main(String[] args) throws IOException {
File file = new File("input.txt");
BufferedReader br = new BufferedReader(new FileReader(file));
Map<String, Integer> wordCount = new HashMap<String, Integer>();
String line;
while ((line = br.readLine()) != null) {
String[] words = line.split("\\s+");
for (String word : words) {
if (wordCount.containsKey(word)) {
wordCount.put(word, wordCount.get(word) + 1);
} else {
wordCount.put(word, 1);
}
}
}
br.close();
for (Map.Entry<String, Integer> entry : wordCount.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
```
请注意,此代码仅仅是一个示例,您可以根据您的实际需求来定制自己的程序。同时也请注意,要处理各种特殊字符和标点符号,以保证输出的结果正确。
阅读全文
相关推荐
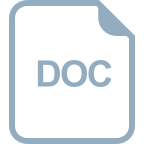
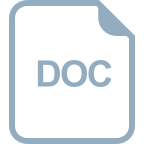
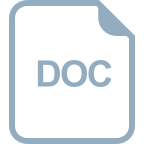
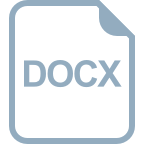






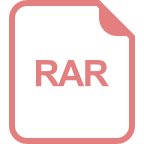







