写一个程序 java统计一个文本文件中单词的个数
时间: 2024-03-06 16:46:26 浏览: 268
可以使用 Java 的 Scanner 和 HashMap 类来实现文本文件中单词的统计。具体步骤如下:
1. 创建一个 Scanner 对象来读取文本文件。
2. 使用 Scanner 的 hasNextLine() 和 nextLine() 方法逐行读取文本文件。
3. 对于每一行文本,使用 String 的 split() 方法按照空格分割单词,并将每个单词加入到 HashMap 中。HashMap 的 key 存储单词,value 存储单词的出现次数。
4. 统计完成后,遍历 HashMap,输出单词及其出现次数。
以下是示例代码:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.HashMap;
import java.util.Scanner;
public class WordCount {
public static void main(String[] args) {
// 打开文件
File file = new File("filename.txt");
Scanner scanner = null;
try {
scanner = new Scanner(file);
} catch (FileNotFoundException e) {
e.printStackTrace();
}
// 统计单词出现次数
HashMap<String, Integer> wordCount = new HashMap<>();
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] words = line.split(" ");
for (String word : words) {
if (wordCount.containsKey(word)) {
wordCount.put(word, wordCount.get(word) + 1);
} else {
wordCount.put(word, 1);
}
}
}
// 输出结果
for (String word : wordCount.keySet()) {
System.out.println(word + ": " + wordCount.get(word));
}
}
}
```
其中,文件名为 "filename.txt",可以替换为实际的文件名。
阅读全文
相关推荐

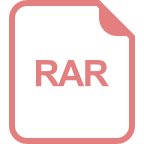



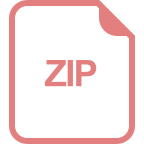











