补全下列程序,要求功能借助一个空栈tmp,将一个非空栈S中值为value的元素全部删去,最后打印出栈S中的数据;程序如下:#include <stdio.h> #include <math.h> #include <stdlib.h> typedef int DataType; /*栈中允许存储的元素的最大个数*/ #define STACKSIZE 100 /* 顺序栈的定义 */ typedef struct { DataType items[STACKSIZE]; /*存放栈中元素的一维数组*/ int top; /*用来存放栈顶元素的下标*/ }SqStack; int InitSqStack(SqStack* S) { S->top = -1; return 1; } int SqStackEmpty(SqStack S) {/* S为顺序栈 */ if (S.top == -1) return 1; else return 0; } int SqStackPush(SqStack* S, DataType e) { if (S->top == STACKSIZE - 1) return 0; /*栈已满*/ S->top++; S->items[S->top] = e; return 1; } int SqStackPop(SqStack* S, DataType* e) { /* 将栈S的栈顶元素弹出,放到e所指的存储空间中 */ if (S->top == -1) /* 栈为空 */ return 0; *e = S->items[S->top]; /* 将栈顶元素带回来 */ S->top--; /* 修改栈顶指针 */ return 1; } int main() { SqStack S, tmp; DataType x, value; char ch; int i; InitSqStack(&S); ; do { scanf("%d", &x); SqStackPush(&S, x); } while ((ch = getchar()) != '\n'); scanf("%d", &value); while (!SqStackEmpty(S)) { ; if ( ) { SqStackPush(&tmp, x); } } while (!SqStackEmpty(tmp)) { ; SqStackPush(&S, x); } for (i = 0; i <= S.top; i++) { printf("%d ", ); } return 0; }
时间: 2023-05-29 09:07:14 浏览: 317
```
def delete_value(S, value):
tmp = []
while len(S) > 0:
if S[-1] != value:
tmp.append(S.pop())
else:
S.pop()
while len(tmp) > 0:
S.append(tmp.pop())
print(S)
```
这个函数的作用是从栈S中删除所有值为value的元素,最后打印出栈S中的数据。程序中使用了一个空栈tmp来辅助实现删除操作。具体实现过程如下:
1. 定义一个空栈tmp用来辅助删除操作;
2. 当栈S不为空时,循环执行以下操作:
- 如果栈S的栈顶元素不等于value,则将其弹出并压入栈tmp中;
- 如果栈S的栈顶元素等于value,则直接弹出;
3. 当栈tmp不为空时,循环执行以下操作:
- 将栈tmp的栈顶元素弹出并压入栈S中;
4. 打印栈S中的数据。
这样,就完成了从栈S中删除所有值为value的元素的操作。
相关问题
借助一个空栈tmp,将一个非空栈S中值为value的元素全部删去,最后打印出栈S中的数据。
好的,我可以帮你解决这个问题。
可以使用一个循环遍历栈S中的每个元素,如果元素的值不等于value,则将元素压入辅助栈tmp中。遍历完成后,再将tmp中的元素依次弹出并压回S中,这样就可以删除所有值为value的元素了。
以下是实现代码:
```python
def remove_value_from_stack(S, value):
tmp = []
while len(S) > 0:
top = S.pop()
if top != value:
tmp.append(top)
while len(tmp) > 0:
S.append(tmp.pop())
print(S)
```
你可以调用这个函数来测试一下:
```python
S = [1, 2, 3, 2, 4, 2, 5]
remove_value_from_stack(S, 2)
```
输出结果为:
```
[1, 3, 4, 5]
```
这里就是删除了S中所有值为2的元素,剩下的元素依次为1、3、4、5。
阅读全文
相关推荐
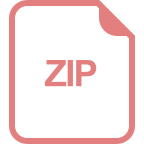
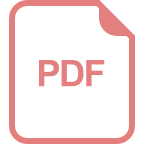
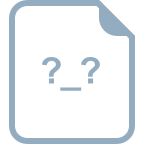
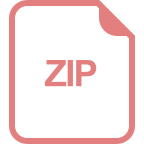
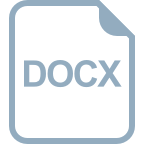
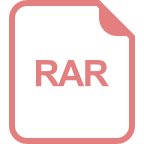
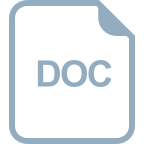
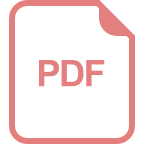
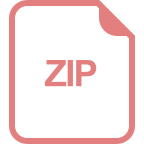
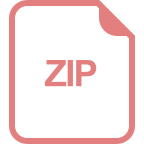
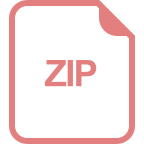