x and y can be no greater than 2D, but have shapes (197,) and (197, 3, 1)
时间: 2024-01-24 22:01:41 浏览: 59
这个错误提示的含义是你的 `x` 和 `y` 数组维度超过了二维。在大多数情况下,数据都是二维的,如 `(n_samples, n_features)` 的形式。但是,如果你在使用某些特定的函数时,数据维度必须是二维或更低(即一维),则会发生此错误。
在你的情况下,`x` 数组的形状为 `(197,)`,即它是一个一维数组,而 `y` 数组的形状为 `(197, 3, 1)`,即它是一个三维数组。因此,你需要将这些数组转换为二维形式。你可以使用 `numpy.reshape()` 函数将一维数组转换为二维形式,例如:
``` python
import numpy as np
# 将 x 转换为二维形式
x = np.reshape(x, (-1, 1))
# 将 y 转换为二维形式
y = np.reshape(y, (len(y), -1))
```
这里,`numpy.reshape()` 函数的第一个参数是要转换的数组,第二个参数是新数组的形状。`-1` 表示该维度的大小应该根据数组的其他维度推断得出。因此,`np.reshape(x, (-1, 1))` 将 `x` 转换为一个二维数组,其中第一维的大小由 `x` 的长度自动推断得出,第二维的大小为1。同样地,`np.reshape(y, (len(y), -1))` 将 `y` 转换为一个二维数组,其中第一维的大小为 `y` 的长度,第二维的大小由 `y` 的其他维度推断得出。
相关问题
解释报错 x and y can be no greater than 2D, but have
该错误表明输入的数据 `x` 和 `y` 的维度不能超过二维。通常情况下,机器学习算法和深度学习模型对输入数据的维度有一定的限制。
可能的原因是输入的数据 `x` 或 `y` 维度超过了二维,而算法或模型只能处理二维数据。这可能是因为数据的形状或结构不符合算法或模型的要求。
为了解决这个问题,你可以检查输入数据的维度,并确保其维度不超过二维。可以使用工具库(如 NumPy 或 Pandas)来查看数据的形状,并相应地对数据进行处理或转换,以使其符合算法或模型的要求。
另外,如果你能提供更多的上下文信息或相关代码,我可以给出更具体的解释和建议。
ValueError: x and y can be no greater than 2D, but have shapes (499,) and (499, 14, 1)
This error occurs when trying to plot 2D data against 3D data using Matplotlib.
In order to fix this error, you need to reshape your 3D data to 2D data. One possible approach is to select a specific dimension of the 3D data to plot against the 2D data. For example, if you have a 3D array with shape (499, 14, 1), you can select the first dimension (499) to plot against the 2D array. Here's an example code snippet:
``` python
import numpy as np
import matplotlib.pyplot as plt
# create some example data
x = np.linspace(0, 1, 499)
y = np.random.randn(499, 14, 1)
# reshape the 3D data to 2D data by selecting the first dimension
y_2d = y[:, 0, 0]
# plot the data
plt.plot(x, y_2d)
plt.show()
```
In this example, we selected the first dimension of the 3D array using `y[:, 0, 0]`, which gives us a 2D array with shape (499,). We can then plot this 2D array against the 1D array `x` using Matplotlib.
相关推荐
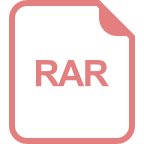
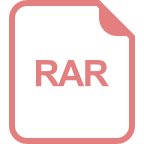












