ValueError: x and y can be no greater than 2D, but have shapes (288,) and (288, 307, 1)
时间: 2024-05-07 08:21:39 浏览: 23
这个错误是由于你正在尝试绘制一个二维图形,但是你提供的数据却是三维的。你可以检查一下你正在使用的绘图函数,看看它需要的数据格式是什么样的,然后将你的数据转换为相应的格式。
比如,如果你正在使用 matplotlib 库中的 plot 函数绘制二维图形,那么它需要的数据格式应该是两个一维数组,分别表示 x 轴和 y 轴上的数据点。而你提供的数据中,x 轴上的数据点是一个一维数组,y 轴上的数据点则是一个三维数组,这显然与 plot 函数需要的数据格式不符。
因此,你需要将 y 轴上的数据点转换为一个一维数组,才能正确地使用 plot 函数。具体的转换方法可能因你的数据格式而异,你可以尝试使用 numpy 库中的 reshape 函数或者 flatten 函数来实现。例如,如果你的 y 轴上的数据点是一个形状为 (288, 307, 1) 的三维数组,你可以使用以下代码将它转换为一个形状为 (288, 307) 的二维数组:
```python
import numpy as np
y_2d = np.reshape(y, (288, 307))
```
或者使用 flatten 函数:
```python
y_2d = y.flatten().reshape(288, 307)
```
然后,你就可以将 x 和 y_2d 作为参数传递给 plot 函数了:
```python
import matplotlib.pyplot as plt
plt.plot(x, y_2d)
plt.show()
```
相关问题
ValueError: x and y can be no greater than 2D, but have shapes (499,) and (499, 14, 1)
This error occurs when trying to plot 2D data against 3D data using Matplotlib.
In order to fix this error, you need to reshape your 3D data to 2D data. One possible approach is to select a specific dimension of the 3D data to plot against the 2D data. For example, if you have a 3D array with shape (499, 14, 1), you can select the first dimension (499) to plot against the 2D array. Here's an example code snippet:
``` python
import numpy as np
import matplotlib.pyplot as plt
# create some example data
x = np.linspace(0, 1, 499)
y = np.random.randn(499, 14, 1)
# reshape the 3D data to 2D data by selecting the first dimension
y_2d = y[:, 0, 0]
# plot the data
plt.plot(x, y_2d)
plt.show()
```
In this example, we selected the first dimension of the 3D array using `y[:, 0, 0]`, which gives us a 2D array with shape (499,). We can then plot this 2D array against the 1D array `x` using Matplotlib.
解释这段错误ValueError: x and y can be no greater than 2D, but have shapes (28789,) and torch.Size([28789, 14, 1])
这个错误 `ValueError: x and y can be no greater than 2D, but have shapes (28789,) and torch.Size([28789, 14, 1])` 表明在某个操作中,输入数据 `x` 和 `y` 的维度超过了二维。通常情况下,这可能是由于数据的形状不符合要求导致的。
根据错误信息,`x` 的形状是 `(28789,)`,表示一个一维数组或向量。而 `y` 的形状是 `torch.Size([28789, 14, 1])`,表示一个三维张量,其中第一维有 28789 个元素。
根据错误提示,期望的形状应该是二维的。因此,需要检查代码中涉及到 `x` 和 `y` 的操作,并确保它们的维度符合要求。
可能的解决方法包括:
1. 确保输入数据的形状正确,并与模型的期望输入形状相匹配。
2. 检查数据预处理或加载过程中是否出现了错误,导致数据的形状不正确。
3. 检查模型定义和前向传播函数,确保输入数据的形状与模型定义的输入层匹配。
如果你能提供更多的上下文信息或相关代码,我可以给出更具体的解释和建议。
相关推荐
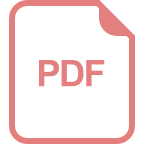
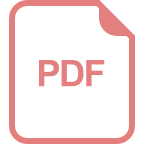
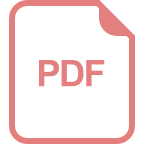












