编写算法,实现下面函数的功能。函数void insert(char*s,char*t,int pos)将字符串t插入到字符串s中,插入位置为pos。假设分配给字符串s的空间足够让字符串t插入。 (说明:不得使用任何库函数)用C语言编写代码 加注释
时间: 2024-03-25 10:36:18 浏览: 29
```c
#include <stdio.h>
// 函数 void insert(char*s,char*t,int pos)将字符串t插入到字符串s中,插入位置为pos。
void insert(char* s, char* t, int pos) {
int t_len = 0;
// 计算字符串 t 的长度
while (t[t_len] != '\0') {
t_len++;
}
int s_len = 0;
// 计算字符串 s 的长度
while (s[s_len] != '\0') {
s_len++;
}
// 从字符串 s 的末尾开始,向后移动 t_len 个字符
for (int i = s_len; i >= pos; i--) {
s[i + t_len] = s[i];
}
// 将字符串 t 插入到字符串 s 中
for (int i = 0; i < t_len; i++) {
s[pos + i] = t[i];
}
}
int main() {
char s[100] = "hello world";
char t[100] = "beautiful ";
int pos = 6;
// 将字符串 t 插入到字符串 s 的 pos 位置
insert(s, t, pos);
printf("%s\n", s); // 输出 hello beautiful world
return 0;
}
```
注释写得详细,主要思路是先计算出字符串 s 和字符串 t 的长度,然后从字符串 s 的末尾开始,向后移动 t_len 个字符,最后将字符串 t 插入到字符串 s 中。
相关推荐
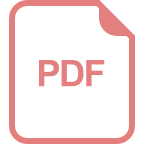
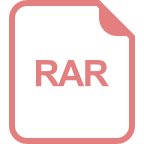
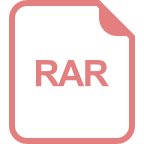
















