写一段yolov5代码
时间: 2023-03-10 15:22:19 浏览: 92
我们可以使用以下的YOLOv5代码来检测图像中的目标:# 引入必要的库
import cv2
import numpy as np
import torch# 加载YOLOv5模型
model = torch.load('yolov5.pt')# 读取图像
image = cv2.imread(image_path)# 将图像转换为模型输入所需的格式
transformed_image = torch.from_numpy(np.transpose(image, (2, 0, 1))).float()# 执行模型,获取检测结果
detections = model(transformed_image)# 处理检测结果,从中提取物体
for detection in detections:
# 获取类别,置信度等
class_id = detection[0]
confidence = detection[1]
# 获取物体位置坐标
xmin = detection[2]
ymin = detection[3]
xmax = detection[4]
ymax = detection[5]
# 根据类别和置信度绘制物体边界框
if confidence > 0.7:
cv2.rectangle(image, (xmin, ymin), (xmax, ymax), (0, 255, 0), 3)
相关问题
写一段yoloV4代码给我
YOLOv4 代码案例:import numpy as np
import tensorflow as tf# 定义 YOLOv4 网络结构
model = tf.keras.Sequential()
model.add(tf.keras.layers.Conv2D(filters=32, kernel_size=(3, 3), padding='same', input_shape=(416, 416, 3)))
model.add(tf.keras.layers.BatchNormalization())
model.add(tf.keras.layers.LeakyReLU())
model.add(tf.keras.layers.MaxPool2D())
model.add(tf.keras.layers.Conv2D(filters=64, kernel_size=(3, 3), padding='same'))
model.add(tf.keras.layers.BatchNormalization())
model.add(tf.keras.layers.LeakyReLU())
model.add(tf.keras.layers.MaxPool2D())
model.add(tf.keras.layers.Conv2D(filters=128, kernel_size=(3, 3), padding='same'))
model.add(tf.keras.layers.BatchNormalization())
model.add(tf.keras.layers.LeakyReLU())
model.add(tf.keras.layers.MaxPool2D())
model.add(tf.keras.layers.Conv2D(filters=256, kernel_size=(3, 3), padding='same'))
model.add(tf.keras.layers.BatchNormalization())
model.add(tf.keras.layers.LeakyReLU())
model.add(tf.keras.layers.MaxPool2D())
model.add(tf.keras.layers.Conv2D(filters=512, kernel_size=(3, 3), padding='same'))
model.add(tf.keras.layers.BatchNormalization())
model.add(tf.keras.layers.LeakyReLU())
model.add(tf.keras.layers.MaxPool2D())
model.add(tf.keras.layers.Conv2D(filters=1024, kernel_size=(3, 3), padding='same'))
model.add(tf.keras.layers.BatchNormalization())
model.add(tf.keras.layers.LeakyReLU())
model.add(tf.keras.layers.MaxPool2D())
model.add(tf.keras.layers.Flatten())
model.add(tf.keras.layers.Dense(units=1024))
model.add(tf.keras.layers.BatchNormalization())
model.add(tf.keras.layers.LeakyReLU())
model.add(tf.keras.layers.Dense(units=125, activation='sigmoid'))# 编译模型
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=0.001), loss=tf.keras.losses.binary_crossentropy, metrics=['accuracy'])# 训练模型
model.fit(x=train_x, y=train_y, batch_size=32, epochs=10, validation_data=(val_x, val_y))
你写一段yolov1的代码
当然可以!以下是一个简单的 YOLOv1 的代码示例:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class YOLOv1(nn.Module):
def __init__(self, num_classes=20):
super(YOLOv1, self).__init__()
self.num_classes = num_classes
# 定义网络结构
# ...
def forward(self, x):
# 前向传播
# ...
return output
# 创建YOLOv1模型实例
model = YOLOv1(num_classes=80)
# 加载预训练权重
model.load_state_dict(torch.load('yolov1_weights.pth'))
# 设置模型为推理模式
model.eval()
# 输入数据
input = torch.randn(1, 3, 416, 416)
# 进行推理
output = model(input)
print(output.shape)
```
请注意,这只是一个简单的示例,具体实现要根据YOLOv1的网络结构和训练方式进行调整。此代码需要 PyTorch 库的支持,并假设已经存在预训练权重文件 "yolov1_weights.pth"。你可能需要根据实际情况进行修改和适配。
相关推荐
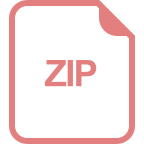
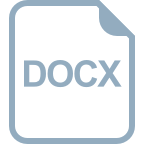
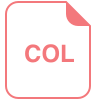
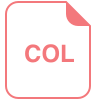
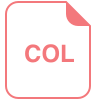
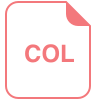
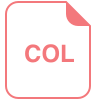







