写一段yolov5的onnxruntime cpu推理代码
时间: 2023-10-24 14:09:01 浏览: 48
以下是一个使用ONNX Runtime在CPU上进行Yolov5推理的Python代码示例:
```python
import cv2
import numpy as np
import onnxruntime as ort
# 加载模型
model_path = "yolov5s.onnx"
session = ort.InferenceSession(model_path)
# 输入和输出节点名称
input_name = session.get_inputs()[0].name
output_names = [session.get_outputs()[i].name for i in range(len(session.get_outputs()))]
# 读取图像
img_path = "test.jpg"
img = cv2.imread(img_path)
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img = cv2.resize(img, (640, 640))
img = img.astype(np.float32) / 255.0
img = np.transpose(img, (2, 0, 1))
img = np.expand_dims(img, axis=0)
# 进行推理
outputs = session.run(output_names, {input_name: img})
# 处理输出结果
boxes = outputs[0][0]
scores = outputs[1][0]
labels = outputs[2][0]
# 可视化结果
for i in range(len(boxes)):
if scores[i] > 0.5:
box = boxes[i]
x1, y1, x2, y2 = box
label = int(labels[i])
color = (0, 255, 0)
cv2.rectangle(img, (x1, y1), (x2, y2), color, 2)
cv2.putText(img, f"{label}", (x1, y1-10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, color, 2)
cv2.imshow("result", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在此示例中,我们首先使用ONNX Runtime加载了已经训练好的Yolov5模型。然后,我们读取了一个测试图像并将其预处理成模型所需的输入格式。最后,我们通过调用`session.run`函数来进行推理,并处理输出结果。最后,我们将结果可视化并显示在屏幕上。
相关推荐
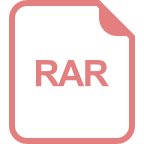
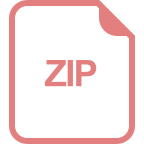
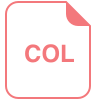
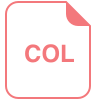
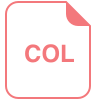
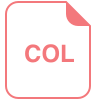
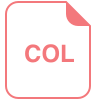









