fstream
时间: 2023-11-09 19:10:34 浏览: 120
`fstream` 是 C++ 标准库中的一个文件流类,用于读写文件。它继承自 `ifstream` 和 `ofstream` 类,可以同时进行读写操作。在使用 `fstream` 之前,需要包含 `<fstream>` 头文件。
以下是 `fstream` 的一些常用操作:
1. 打开文件
`open()` 函数用于打开文件,它有两个参数:文件名和文件打开模式。文件打开模式可以是输入模式、输出模式或输入输出模式。常用的文件打开模式有:
- `ios::in` :以输入方式打开文件
- `ios::out` :以输出方式打开文件
- `ios::app` :以输出方式打开文件,并将写入位置移动到文件末尾
- `ios::binary` :以二进制模式打开文件
示例代码:
```cpp
#include <fstream>
using namespace std;
int main() {
fstream file;
file.open("example.txt", ios::in | ios::out);
if (!file.is_open()) {
cout << "Failed to open file." << endl;
return 0;
}
// 文件操作...
file.close();
return 0;
}
```
2. 读取文件
`getline()` 函数用于按行读取文件,它有两个参数:字符数组和读取字符数。如果读取成功,返回值为字符数组,否则返回空指针。示例代码:
```cpp
char buffer[256];
while (file.getline(buffer, 256)) {
cout << buffer << endl;
}
```
3. 写入文件
`<<` 运算符用于向文件写入数据。示例代码:
```cpp
file << "Hello, world!" << endl;
```
4. 获取文件指针位置
`tellg()` 和 `tellp()` 函数分别返回文件输入流和输出流当前位置的指针位置。示例代码:
```cpp
cout << "Input position: " << file.tellg() << endl;
cout << "Output position: " << file.tellp() << endl;
```
5. 移动文件指针位置
`seekg()` 和 `seekp()` 函数分别用于移动文件输入流和输出流的指针位置。示例代码:
```cpp
file.seekg(0, ios::beg); // 移动到文件开头
file.seekp(0, ios::end); // 移动到文件末尾
```
6. 关闭文件
`close()` 函数用于关闭文件流。示例代码:
```cpp
file.close();
```
阅读全文
相关推荐



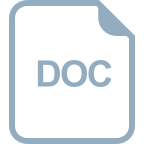














