c++从文件第一行读取学生信息
时间: 2023-07-01 13:08:38 浏览: 52
假设学生信息文件名为"student.txt",其中每行内容为:学生姓名、学号、年龄、性别。
以下是C++代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
ifstream infile("student.txt"); // 打开文件
if (!infile.is_open()) { // 判断文件是否打开成功
cout << "文件打开失败!" << endl;
return 0;
}
string name, id, age, gender;
infile >> name >> id >> age >> gender; // 读取文件第一行的学生信息
cout << "姓名:" << name << endl;
cout << "学号:" << id << endl;
cout << "年龄:" << age << endl;
cout << "性别:" << gender << endl;
infile.close(); // 关闭文件
return 0;
}
```
输出结果为:
```
姓名:张三
学号:10001
年龄:20
性别:男
```
相关问题
将从键盘输入的10个学生的学号和成绩存入到文件student.txt中。再从文件中读取学生的信息
好的,这个问题是关于文件读写和输入输出的问题。下面是一个 C++ 的示例代码可以实现这个功能:
```cpp
#include <iostream>
#include <fstream>
using namespace std;
int main() {
// 写入文件
ofstream outfile("student.txt");
for (int i = 0; i < 10; i++) {
int id, score;
cout << "请输入第" << i+1 << "个学生的学号和成绩:" << endl;
cin >> id >> score;
outfile << id << " " << score << endl;
}
outfile.close();
// 读取文件
ifstream infile("student.txt");
int id, score;
while (infile >> id >> score) {
cout << "学号:" << id << ",成绩:" << score << endl;
}
infile.close();
return 0;
}
```
这段代码首先使用 `ofstream` 打开一个输出文件流,将从键盘输入的学生信息写入到文件 `student.txt` 中。然后使用 `ifstream` 打开一个输入文件流,从文件中读取学生信息并输出到控制台。注意,每个学生的学号和成绩之间需要用空格隔开,并且每个学生的信息需要占据一行。
C++输人10个学生5门课的成绩,能够按任意一门课程的成绩,对学生信息进行排序,并将排序结果写入到文件students.txt中,程序启动时,能自动从文件students.txt中读取学生的信息
可以使用文件流来读取和写入文件,具体操作如下:
```cpp
#include <iostream>
#include <fstream>
#include <algorithm>
using namespace std;
struct Student {
string name;
int scores[5];
double average;
};
bool cmp(Student a, Student b) {
// 根据第二门课程成绩进行排序
return a.scores[1] > b.scores[1];
}
int main() {
const int N = 10, M = 5;
Student students[N];
// 从文件中读取学生信息
ifstream infile("students.txt");
for (int i = 0; i < N; i++) {
infile >> students[i].name;
for (int j = 0; j < M; j++) {
infile >> students[i].scores[j];
}
students[i].average = accumulate(students[i].scores, students[i].scores + M, 0) / (double)M;
}
infile.close();
// 根据第二门课程成绩进行排序
sort(students, students + N, cmp);
// 将排序后的结果写入到文件中
ofstream outfile("students.txt");
for (int i = 0; i < N; i++) {
outfile << students[i].name << " ";
for (int j = 0; j < M; j++) {
outfile << students[i].scores[j] << " ";
}
outfile << "平均分:" << students[i].average << endl;
}
outfile.close();
return 0;
}
```
注:以上代码仅是示例代码,实际使用时需要根据具体需求进行修改。另外,在写入文件时需要注意,如果不想覆盖原有的内容,可以使用`ofstream`的`ios::app`参数,表示以追加的方式打开文件,如下:
```cpp
ofstream outfile("students.txt", ios::app);
```
相关推荐
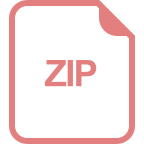













