将3名学生的学号、姓名和成绩写入二进制文件studentinfo.dat中,再将第2名学生的成绩改为627,最后从文件中读取第m(m的值由用户从键盘输入)名学生的信息并将其输出到屏幕上。用c++解决
时间: 2024-03-22 20:42:56 浏览: 77
以下是用C++编写的程序,实现将学生信息写入二进制文件、修改学生信息并读取指定学生信息并输出到屏幕上:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
struct Student {
int id;
string name;
float score;
};
int main() {
// 创建结构体数组并初始化
Student students[3] = {
{ 1001, "Tom", 85.5 },
{ 1002, "Jerry", 90.0 },
{ 1003, "Mickey", 95.5 }
};
// 将学生信息写入二进制文件
ofstream outfile("studentinfo.dat", ios::binary);
if (!outfile) {
cout << "Failed to open file!" << endl;
return 0;
}
outfile.write((char*)students, sizeof(Student) * 3);
outfile.close();
// 修改学生信息
students[1].score = 627.0;
// 读取指定学生的信息并输出到屏幕上
int m;
cout << "Please input the index of the student you want to read: ";
cin >> m;
Student read_student;
ifstream infile("studentinfo.dat", ios::binary);
if (!infile) {
cout << "Failed to open file!" << endl;
return 0;
}
infile.seekg(sizeof(Student) * (m-1), ios::beg);
infile.read((char*)&read_student, sizeof(Student));
infile.close();
cout << "Student " << m << endl;
cout << "ID: " << read_student.id << endl;
cout << "Name: " << read_student.name << endl;
cout << "Score: " << read_student.score << endl;
return 0;
}
```
程序首先创建了一个包含3名学生信息的结构体数组,并将其写入二进制文件`studentinfo.dat`中。然后程序修改了第2名学生的成绩为627,并从文件中读取第m(由用户从键盘输入)名学生的信息并输出到屏幕上。
注意,在读取指定学生信息时,程序使用了`seekg`函数将文件指针移动到指定位置。同时,读取的字节数为一个结构体的大小。
阅读全文
相关推荐
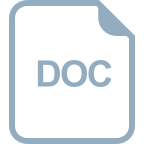
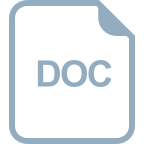
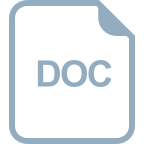




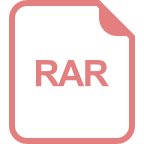






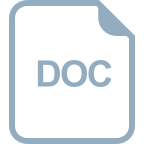
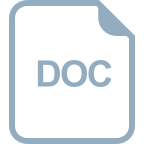
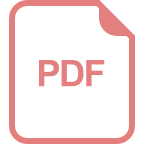