编写并测试程序:(输入输出流的write、read函数程序) 首先将3名学生的学号、姓名和成绩写入二进制文件studentinfo.dat中,然后将该文件中的数据读到结构体数组中,并将其输出到屏幕上。用c++解决
时间: 2024-03-22 19:42:51 浏览: 17
以下是用C++编写的程序,实现将学生信息写入二进制文件,并将其读取并输出到屏幕上:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
struct Student {
int id;
string name;
float score;
};
int main() {
// 创建结构体数组并初始化
Student students[3] = {
{ 1001, "Tom", 85.5 },
{ 1002, "Jerry", 90.0 },
{ 1003, "Mickey", 95.5 }
};
// 将学生信息写入二进制文件
ofstream outfile("studentinfo.dat", ios::binary);
if (!outfile) {
cout << "Failed to open file!" << endl;
return 0;
}
outfile.write((char*)students, sizeof(Student) * 3);
outfile.close();
// 读取二进制文件中的学生信息到结构体数组中,并输出到屏幕上
Student read_students[3];
ifstream infile("studentinfo.dat", ios::binary);
if (!infile) {
cout << "Failed to open file!" << endl;
return 0;
}
infile.read((char*)read_students, sizeof(Student) * 3);
infile.close();
for (int i = 0; i < 3; i++) {
cout << "Student " << i+1 << endl;
cout << "ID: " << read_students[i].id << endl;
cout << "Name: " << read_students[i].name << endl;
cout << "Score: " << read_students[i].score << endl;
}
return 0;
}
```
程序首先创建了一个包含3名学生信息的结构体数组,并将其写入二进制文件`studentinfo.dat`中。然后程序读取该文件中的数据到另一个结构体数组中,并将其输出到屏幕上。
注意,程序中的`ofstream`和`ifstream`类分别用于写入和读取二进制文件。同时,`ios::binary`参数指定文件以二进制模式打开。在读写二进制文件时,需要将结构体强制转换为`char*`类型,并指定读写的字节数。
相关推荐
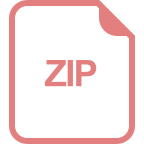
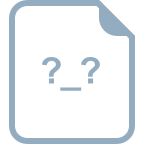















