使用c plus plus写出满足下列要求的代码:重用上题的Student类,再定义一个StudentArray类,含有Student*和学生人数n两个数据成员,定义相应的构造函数和析构函数完成学生数组对象的创建和释放,再编写3个成员函数分别完成以下功能: (1)录入n个学生信息(n在主函数中由用户输入); (2)输出n个学生信息; (3)将n个学生信息保存到文件student.dat中(要求使用二进制保存,使用write()函数); (4)按学号查找学生信息,其中所有学生信息都从文件student.dat中获取(也可以分别定义两个函数完成,一个从文件读信息,一个从数组中查找信息)。 在主函数中定义学生数组对象对上述函数进行测试。
时间: 2023-03-10 20:16:52 浏览: 213
使用 C++ 编写代码实现上述要求,示例代码如下:// 定义 Student 类
class Student
{
public:
int num;
char name[20];
int score;
};// 定义 StudentArray 类
class StudentArray
{
private:
Student* pStu; // 学生指针
int n; // 学生人数
public:
// 构造函数
StudentArray(int n):n(n)
{
pStu = new Student[n]; // 动态分配 n 个 Student 对象
}
// 析构函数
~StudentArray()
{
delete[] pStu; // 释放 pStu 所指向的内存
}
// 录入 n 个学生信息
void Input()
{
for (int i = 0; i < n; i++)
{
cout << "请输入第 " << i+1 << " 个学生的学号、姓名和成绩:" << endl;
cin >> pStu[i].num >> pStu[i].name >> pStu[i].score;
}
}
// 输出 n 个学生信息
void Output()
{
for (int i = 0; i < n; i++)
{
cout << "第 " << i+1 << " 个学生的学号、姓名和成绩:" << endl;
cout << pStu[i].num << " " << pStu[i].name << " " << pStu[i].score << endl;
}
}
// 将 n 个学生信息保存到文件 student.dat 中
void Save()
{
ofstream outFile("student.dat", ios::binary); // 以二进制的方式打开文件
if (!outFile)
{
cout << "打开文件失败!" << endl;
return;
}
outFile.write((char*)pStu, n*sizeof(Student)); // 将 pStu 所指向的内存写入文件
outFile.close(); // 关闭文件
}
// 按学号查找学生信息
int Find(int num)
{
// 从文件中读取 n 个学生信息
ifstream inFile("student.dat", ios::binary);
if (!inFile)
{
cout << "打开文件失败!" << endl;
return -1;
}
inFile.read((char*)pStu, n*sizeof(Student)); // 从文件中读取 n 个学生对象
inFile.close(); // 关闭文件 // 从数组中查找学生信息
for (int i = 0; i < n; i++)
{
if (pStu[i].num == num)
{
cout << "找到该学生信息:";
cout << pStu[i].num << " " << pStu[i].name << " " << pStu[i].score << endl;
return i; // 查找成功,返回学生索引
}
}
return -1; // 查找失败,返回-1
}
};int main()
{
int n;
cout << "请输入学生人数:";
cin >> n;
StudentArray stuArr(n); // 定义学生数组对象
stuArr.Input(); // 录入 n 个学生信息
stuArr.Output(); // 输出 n 个学生信息
stuArr.Save(); // 将 n 个学生信息保存到文件 student.dat 中
int num;
cout << "请输入要查找的学生学号:";
cin >> num;
int index = stuArr.Find(num); // 按学号查找学生信息
if (index == -1)
cout << "未找到该学生信息!" << endl;
return 0;
}
阅读全文
相关推荐
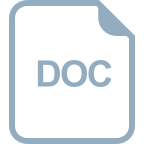
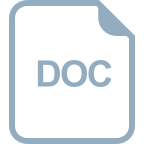
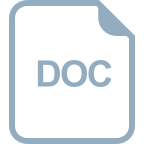
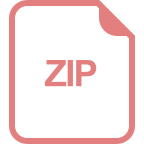
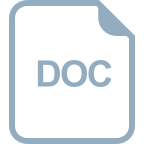
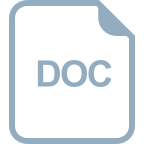
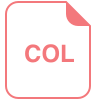
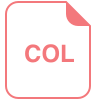
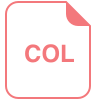
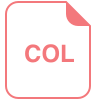
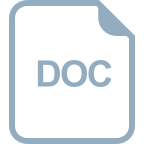
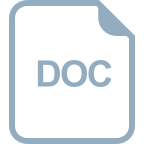
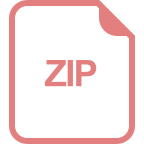