将键盘输入的学生信息(包括学号 姓名和成绩)逐行写入文件Student.dat,当输入exit时结束。 统计文件 Student.dat中包含的学生数,以及平均分、最高分和最低分。 程序检查成绩输入值,如果不是数值信息,或是负的数值信息,或超过成绩范围 (100),抛出相应的异常。在抛出异常之前应提供详细的信息说明,从而使使 用该程序的用户了解所出现的问题,同时考虑上述异常出现时程序应该如何处理。
时间: 2024-03-02 19:52:09 浏览: 27
好的,下面是Java程序的实现思路:
1. 使用while循环,不断读取键盘输入的学生信息,直到输入exit为止。
2. 将每个学生的信息逐行写入Student.dat文件中,使用文件流实现。
3. 使用try-catch语句捕获可能出现的异常情况,如输入非数字字符、输入负数或超出成绩范围等。
4. 在出现异常时,使用throw语句抛出相应的异常,并提供详细的错误信息。
5. 统计文件中包含的学生数、平均分、最高分和最低分,使用文件流读取Student.dat文件中的数据,计算出相应的统计结果,并输出到屏幕上。
下面是Java代码实现:
```java
import java.io.*;
class ScoreOutOfRangeException extends Exception {
private int score;
public ScoreOutOfRangeException(int score) {
this.score = score;
}
public String toString() {
return "成绩超出范围: " + score;
}
}
class InvalidScoreException extends Exception {
private String score;
public InvalidScoreException(String score) {
this.score = score;
}
public String toString() {
return "无效的成绩: " + score;
}
}
public class Main {
public static void main(String[] args) {
try {
FileWriter writer = new FileWriter("Student.dat");
BufferedWriter out = new BufferedWriter(writer);
int count = 0;
int totalScore = 0;
int maxScore = -1;
int minScore = 101;
BufferedReader in = new BufferedReader(new InputStreamReader(System.in));
while (true) {
System.out.print("请输入学生信息(学号 姓名 成绩): ");
String inputStr = in.readLine();
if (inputStr.equals("exit")) {
break;
}
String[] studentInfo = inputStr.split(" ");
if (studentInfo.length != 3) {
throw new IllegalArgumentException("输入格式错误");
}
int score = Integer.parseInt(studentInfo[2]);
if (score < 0 || score > 100) {
throw new ScoreOutOfRangeException(score);
}
count++;
totalScore += score;
maxScore = Math.max(maxScore, score);
minScore = Math.min(minScore, score);
out.write(inputStr + "\n");
}
out.close();
if (count > 0) {
System.out.println("学生数: " + count);
System.out.println("平均分: " + (double) totalScore / count);
System.out.println("最高分: " + maxScore);
System.out.println("最低分: " + minScore);
}
} catch (IOException e) {
System.err.println("IOException: " + e.getMessage());
} catch (NumberFormatException e) {
System.err.println("成绩格式错误: " + e.getMessage());
} catch (ScoreOutOfRangeException e) {
System.err.println(e.toString());
} catch (InvalidScoreException e) {
System.err.println(e.toString());
} catch (Exception e) {
System.err.println("Exception: " + e.getMessage());
}
}
}
```
在这个程序中,我们定义了两个异常类:ScoreOutOfRangeException和InvalidScoreException,分别用于处理成绩超出范围和无效成绩的情况。在try-catch语句中,我们捕获可能出现的异常情况,并使用throw语句抛出相应的异常,并在异常信息中提供详细的错误信息。在程序运行结束后,我们使用文件流读取Student.dat文件中的数据,计算出相应的统计结果,并输出到屏幕上。
希望我的回答能对你有所帮助!
相关推荐
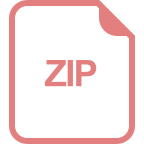
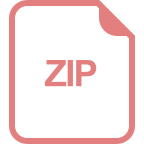















