java发送邮件带附件
时间: 2023-10-03 08:06:58 浏览: 30
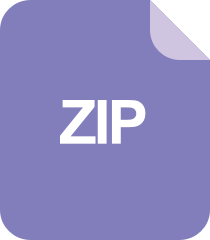
java 邮件发送 可带附件
好的,以下是 Java 发送带附件的邮件的示例代码:
```java
import java.util.Properties;
import javax.mail.Authenticator;
import javax.mail.Message;
import javax.mail.PasswordAuthentication;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import javax.mail.internet.MimeUtility;
public class SendEmailWithAttachment {
public static void main(String[] args) throws Exception {
// 邮件配置
String host = "smtp.163.com"; // SMTP 服务器地址
int port = 25; // SMTP 服务器端口
String username = "your_email@163.com"; // 发件人邮箱账号
String password = "your_email_password"; // 发件人邮箱密码
String from = "your_email@163.com"; // 发件人邮箱地址
String to = "recipient_email@example.com"; // 收件人邮箱地址
String subject = "邮件主题";
String text = "邮件正文";
// 创建邮件会话
Properties props = new Properties();
props.put("mail.smtp.host", host);
props.put("mail.smtp.port", port);
props.put("mail.smtp.auth", "true");
Session session = Session.getDefaultInstance(props, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
// 创建邮件消息
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress(from));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(to));
message.setSubject(subject);
// 创建邮件正文
MimeBodyPart textPart = new MimeBodyPart();
textPart.setContent(text, "text/plain;charset=UTF-8");
// 创建邮件附件
MimeBodyPart attachmentPart = new MimeBodyPart();
String attachmentFilePath = "attachment.txt"; // 附件文件路径
attachmentPart.attachFile(attachmentFilePath);
attachmentPart.setFileName(MimeUtility.encodeText(attachmentPart.getFileName()));
// 创建邮件多部分内容
MimeMultipart multipart = new MimeMultipart();
multipart.addBodyPart(textPart);
multipart.addBodyPart(attachmentPart);
message.setContent(multipart);
// 发送邮件
Transport.send(message);
System.out.println("邮件发送成功");
}
}
```
其中,需要将 `host`、`port`、`username`、`password`、`from`、`to`、`subject`、`text` 和 `attachmentFilePath` 替换为实际的值。
需要注意的是,附件文件路径需要使用绝对路径,并且需要保证附件文件存在。另外,为了避免中文文件名乱码,需要使用 `MimeUtility.encodeText()` 方法对附件文件名进行编码。
阅读全文
相关推荐
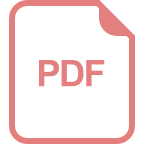














