go 语言http 实现源代码讲解
时间: 2023-11-28 08:04:23 浏览: 53
HTTP是一种基于请求-响应模式的协议,用于客户端和服务器之间的通信。在Go语言中,通过使用标准库中的`net/http`包来实现HTTP服务器和客户端。
HTTP服务器的实现主要包括以下几个步骤:
1. 创建一个HTTP服务器:使用`http.NewServeMux()`函数创建一个HTTP请求路由器。
```go
mux := http.NewServeMux()
```
2. 定义HTTP处理函数:定义处理HTTP请求的函数,这些函数需要实现`http.Handler`接口,即拥有`ServeHTTP(ResponseWriter, *Request)`方法。
```go
func helloHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, World!")
}
```
3. 绑定HTTP处理函数:使用`mux.Handle(pattern, handler)`函数将HTTP请求路由器与HTTP处理函数绑定。
```go
mux.Handle("/", http.HandlerFunc(helloHandler))
```
4. 启动HTTP服务器:使用`http.ListenAndServe(addr, handler)`函数启动HTTP服务器,其中`addr`是服务器监听的地址,`handler`是HTTP请求路由器。
```go
http.ListenAndServe(":8080", mux)
```
完整的HTTP服务器实现代码如下:
```go
package main
import (
"fmt"
"net/http"
)
func helloHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, World!")
}
func main() {
mux := http.NewServeMux()
mux.Handle("/", http.HandlerFunc(helloHandler))
http.ListenAndServe(":8080", mux)
}
```
HTTP客户端的实现主要包括以下几个步骤:
1. 创建一个HTTP客户端:使用`http.Client`结构体创建一个HTTP客户端对象。
```go
client := &http.Client{}
```
2. 创建一个HTTP请求:使用`http.NewRequest(method, url, body)`函数创建一个HTTP请求对象,其中`method`是HTTP请求方法,`url`是请求的URL,`body`是请求的消息体。
```go
req, err := http.NewRequest(http.MethodGet, "http://example.com", nil)
```
3. 发送HTTP请求:使用HTTP客户端的`Do(req *http.Request)`方法发送HTTP请求,并返回HTTP响应对象。
```go
resp, err := client.Do(req)
```
4. 处理HTTP响应:使用HTTP响应对象的方法处理HTTP响应,如读取响应内容、获取响应头等。
完整的HTTP客户端实现代码如下:
```go
package main
import (
"fmt"
"io/ioutil"
"net/http"
)
func main() {
client := &http.Client{}
req, err := http.NewRequest(http.MethodGet, "http://example.com", nil)
if err != nil {
panic(err)
}
resp, err := client.Do(req)
if err != nil {
panic(err)
}
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
fmt.Println(string(body))
}
```
相关推荐
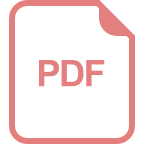
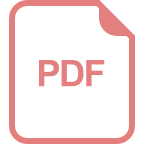
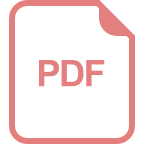
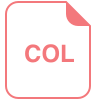
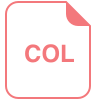
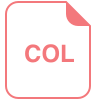
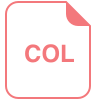
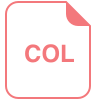









