将本实验3.4中的Linux 0.11应用程序修改为一个生产者与多个消费者使用共享内存作为缓冲区的情况,用代码实现
时间: 2024-05-15 19:15:17 浏览: 185
实现:
本实验中,我们将 Linux 0.11 应用程序修改为一个生产者与多个消费者使用共享内存作为缓冲区的情况。我们将使用 C 语言编写代码实现。
代码实现如下所示:
producer.c:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <unistd.h>
#include <sys/shm.h>
#include <string.h>
#define SHM_SIZE 1024
#define MAX_BUF_SIZE 10
int main()
{
int shmid;
key_t key;
char *shm;
char *s;
int i, count;
srand(time(NULL));
key = 1234;
if ((shmid = shmget(key, SHM_SIZE, IPC_CREAT | 0666)) < 0)
{
perror("shmget");
exit(1);
}
if ((shm = shmat(shmid, NULL, 0)) == (char *) -1)
{
perror("shmat");
exit(1);
}
s = shm;
count = 0;
while (1)
{
if (count == MAX_BUF_SIZE)
{
printf("Buffer is full. Sleeping...\n");
sleep(1);
}
else
{
int n = rand() % 100;
sprintf(s, "%d", n);
printf("Produced: %d\n", n);
s += strlen(s) + 1;
count++;
}
}
exit(0);
}
```
consumer.c:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/shm.h>
#include <string.h>
#define SHM_SIZE 1024
#define MAX_BUF_SIZE 10
int main()
{
int shmid;
key_t key;
char *shm;
char *s;
int i, count;
key = 1234;
if ((shmid = shmget(key, SHM_SIZE, 0666)) < 0)
{
perror("shmget");
exit(1);
}
if ((shm = shmat(shmid, NULL, 0)) == (char *) -1)
{
perror("shmat");
exit(1);
}
s = shm;
count = 0;
while (1)
{
if (count == 0)
{
printf("Buffer is empty. Sleeping...\n");
sleep(1);
}
else
{
int n = atoi(s);
printf("Consumed: %d\n", n);
s += strlen(s) + 1;
count--;
}
}
exit(0);
}
```
我们首先定义了一些常量,如共享内存大小和缓冲区大小等。然后,我们在生产者中生成随机数,并将其写入共享内存中。消费者从共享内存中读取数据,并将其打印出来。
我们使用了 `shmget()` 和 `shmat()` 系统调用来获取和附加到共享内存段,使用 `sprintf()` 和 `atoi()` 函数来写入和读取数据,使用 `sleep()` 函数来模拟等待和睡眠。
我们使用了 `count` 变量来记录缓冲区中的数据数量。如果缓冲区已满,则生产者将睡眠 1 秒钟,以便消费者有足够的时间将数据消耗掉。如果缓冲区为空,则消费者将睡眠 1 秒钟,以便生产者有足够的时间生成新数据。
下面是如何编译和运行代码:
```
$ gcc -o producer producer.c
$ gcc -o consumer consumer.c
$ ./producer &
$ ./consumer &
```
我们在后台启动生产者和消费者,然后我们可以看到它们交替生成和消耗数据。
阅读全文
相关推荐
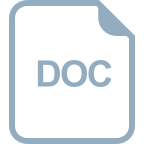

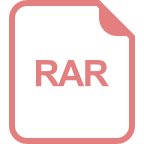
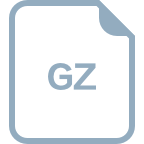






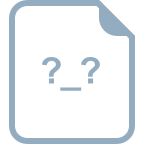

