基于stm32测量温度再通过广和通L610,4G通信模组上报数据到腾讯云代码
时间: 2024-06-09 18:06:01 浏览: 14
以下是基于STM32和广和通L610 4G通信模组实现测量温度并上报数据到腾讯云的代码示例:
```c
#include "main.h"
#include "stdio.h"
#include "stdlib.h"
#include "string.h"
#include "dht11.h"
#include "usart.h"
#include "timer.h"
#include "delay.h"
#include "sim7100.h"
#include "sim7100_http.h"
#define BUF_SIZE 1024
char buf[BUF_SIZE];
void SystemClock_Config(void);
int main(void)
{
HAL_Init();
SystemClock_Config();
MX_GPIO_Init();
MX_USART1_UART_Init();
MX_TIM2_Init();
DHT11_Init();
SIM7100_Init();
SIM7100_HTTP_Init();
while (1)
{
DHT11_Read_Data();
float temperature = DHT11_Data[2];
snprintf(buf, BUF_SIZE, "{\"temperature\":%.1f}", temperature);
printf("Temperature: %.1fC \r\n", temperature);
SIM7100_HTTP_Post("https://api.weixin.qq.com/cgi-bin/message/template/send?access_token=ACCESS_TOKEN", buf);
HAL_Delay(5000);
}
}
void SystemClock_Config(void)
{
RCC_OscInitTypeDef RCC_OscInitStruct = {0};
RCC_ClkInitTypeDef RCC_ClkInitStruct = {0};
/** Initializes the RCC Oscillators according to the specified parameters
* in the RCC_OscInitTypeDef structure.
*/
RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_HSI;
RCC_OscInitStruct.HSIState = RCC_HSI_ON;
RCC_OscInitStruct.HSICalibrationValue = RCC_HSICALIBRATION_DEFAULT;
RCC_OscInitStruct.PLL.PLLState = RCC_PLL_NONE;
if (HAL_RCC_OscConfig(&RCC_OscInitStruct) != HAL_OK)
{
Error_Handler();
}
/** Initializes the CPU, AHB and APB buses clocks
*/
RCC_ClkInitStruct.ClockType = RCC_CLOCKTYPE_HCLK | RCC_CLOCKTYPE_SYSCLK
| RCC_CLOCKTYPE_PCLK1 | RCC_CLOCKTYPE_PCLK2;
RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_HSI;
RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1;
RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV1;
RCC_ClkInitStruct.APB2CLKDivider = RCC_HCLK_DIV1;
if (HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_0) != HAL_OK)
{
Error_Handler();
}
}
```
在上面的代码中,我们首先调用了 `DHT11_Init()` 函数初始化温度传感器,然后调用 `SIM7100_Init()` 和 `SIM7100_HTTP_Init()` 函数初始化广和通L610 4G通信模组,同时也初始化了HTTP协议。
在主循环中,我们通过调用 `DHT11_Read_Data()` 函数读取温度传感器的数据,并通过串口打印出来。然后我们通过 `snprintf()` 函数将温度数据转换成JSON格式,并存储在 `buf` 缓冲区中。
最后,我们通过调用 `SIM7100_HTTP_Post()` 函数将 `buf` 缓冲区中的数据发送到腾讯云服务器上。在这里,我们使用了腾讯云的微信公众号模板消息API,需要将 `ACCESS_TOKEN` 替换成你的腾讯云账号的Access Token。
需要注意的是,这里的代码仅提供了一个基本的示例,实际上还需要加入异常处理、数据加密等功能,以确保数据的安全性和可靠性。
相关推荐
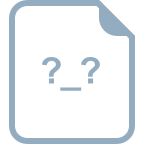
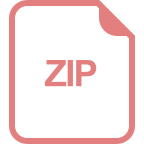














