输入2个字符串,比较它们的大小。要求定义和调用函数cmp(s, t),该函数逐个比较字符串s和t中的对应字符,直到对应字符不等或比较到串尾。若s和t相等则返回0,若不相等则返回不相等字符的差值,即若s大于t则返回一个正数,若s小于t则返回一个负数。输入输出示例如下:# include <stdio.h> #define MAXS 80 int cmp ( char *s, char *t ); int main( ) { char s[MAXS], t[MAXS]; scanf ( "%s%s", s, t ); if ( cmp(s, t) > 0) printf("\"%s\" > \"%s\"\n", s, t); else if ( cmp(s, t) == 0) printf("\"%s\" = \"%s\"\n", s, t); else printf("\"%s\" < \"%s\"\n", s, t); } int cmp ( char *s, char *t ) { while ( ){ if ( *s != *t ) break; ; } return ; }
时间: 2024-02-13 07:02:41 浏览: 259
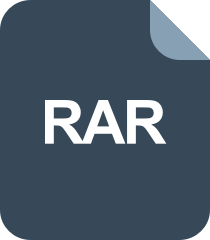
汇编语言字符串比较
好的,下面是实现这个功能的代码:
```
#include <stdio.h>
#define MAXS 80
int cmp(char *s, char *t);
int main() {
char s[MAXS], t[MAXS];
scanf("%s%s", s, t);
if (cmp(s, t) > 0)
printf("\"%s\" > \"%s\"\n", s, t);
else if (cmp(s, t) == 0)
printf("\"%s\" = \"%s\"\n", s, t);
else
printf("\"%s\" < \"%s\"\n", s, t);
return 0;
}
int cmp(char *s, char *t) {
while (*s != '\0' && *t != '\0') {
if (*s != *t) {
break;
}
s++;
t++;
}
return *s - *t;
}
```
这个程序定义了一个名为 `cmp` 的函数,它接收两个指向字符串的指针。在函数中,我们使用一个循环逐个比较字符串 `s` 和 `t` 中的对应字符,直到对应字符不相等或比较到字符串末尾。如果字符串 `s` 大于字符串 `t`,则返回一个正数;如果相等,则返回 0;如果小于,则返回一个负数。
在 `main` 函数中,我们声明了两个字符数组 `s` 和 `t`,并使用 `scanf` 函数从标准输入中读取了它们的值。然后,我们调用了 `cmp` 函数,将指向 `s` 和 `t` 的指针传递给了它。最后,我们根据 `cmp` 函数的返回值打印出相应的比较结果。
阅读全文
相关推荐
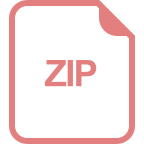
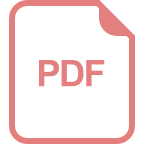















