def encrypte(self): with open('keruan.pth', 'rb') as file: content = file.read() key = 0xAB decrypted_data = bytearray() for byte in content: decrypted_data.append(byte ^ key) decrypted_content_memory = io.BytesIO(decrypted_data) decrypted_content_memory.seek(0) return decrypted_content_memory 优化这段代码
时间: 2024-02-14 07:11:03 浏览: 41
首先,这段代码实现了简单的异或加密解密算法,对文件进行了加密。但是代码中存在一些可以优化的地方。
1. 使用 with 语句自动关闭文件,可以避免忘记关闭文件带来的问题。
2. 可以使用 bytearray 的推导式来简化代码。
3. 可以使用 io.BytesIO 的 from_bytes 方法来将字节数据转换为内存中的二进制数据流,不需要使用 bytearray 作为中间变量。
优化后的代码如下所示:
```
import io
def encrypte(self):
key = 0xAB
with open('keruan.pth', 'rb') as file:
content = file.read()
decrypted_data = bytearray(byte ^ key for byte in content)
decrypted_content_memory = io.BytesIO(decrypted_data)
decrypted_content_memory.seek(0)
return decrypted_content_memory
```
这样可以使代码更加简洁、易读,并且在效率上也不会有太大的影响。
相关问题
def decrypt(): key = 0xAB with open('keruan.pth', 'rb') as file: decrypted_data = bytearray(byte ^ key for byte in file.read()) decrypted_content_memory = io.BytesIO(decrypted_data) decrypted_content_memory.seek(0) return decrypted_content_memory当pysimpelgui窗口关闭时释放这些内存
为了释放这些内存,你可以在 `pysimplegui` 窗口关闭时添加一个回调函数。回调函数可以调用 `decrypt()` 函数并释放其返回的内存。以下是一个示例代码:
```python
import PySimpleGUI as sg
def decrypt():
key = 0xAB
with open('keruan.pth', 'rb') as file:
decrypted_data = bytearray(byte ^ key for byte in file.read())
decrypted_content_memory = io.BytesIO(decrypted_data)
decrypted_content_memory.seek(0)
return decrypted_content_memory
def close_window_callback():
decrypted_content_memory.close()
layout = [[sg.Text('Hello World!')]]
window = sg.Window('My Window', layout, finalize=True)
window.close_callback = close_window_callback
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
decrypted_content_memory = decrypt()
# Do something with decrypted_content_memory
window.close()
```
在上述代码中,我们定义了一个 `close_window_callback()` 函数,它会在 `pysimplegui` 窗口关闭时被调用。在主循环中,我们将这个回调函数赋值给 `window.close_callback` 属性。这样,当用户关闭窗口时,`close_window_callback()` 函数就会自动被调用。
在 `close_window_callback()` 函数中,我们调用了 `decrypted_content_memory.close()` 方法来释放内存。要注意的是,如果你在其他地方也使用了 `decrypted_content_memory`,就不能在这里直接将它赋值为 `None`,否则会导致其他部分出错。
def set_values(self, values): with open('keruan.pth', 'rb') as file: content = file.read() key = 0xAB decrypted_data = bytearray() for byte in content: decrypted_data.append(byte ^ key) decrypted_content_memory = io.BytesIO(decrypted_data) decrypted_content_memory.seek(0) self.config.pth_path = decrypted_content_memory self.config.hubert_path = os.path.join(current_dir, 'hubert_base.pt') self.config.index_path = os.path.join(current_dir, 'keruan.index') self.set_devices(values["sg_input_device"], values["sg_output_device"]) self.config.threhold = values["threhold"] self.config.pitch = values["pitch"] self.config.block_time = values["block_time"] self.config.crossfade_time = values["crossfade_length"] self.config.extra_time = values["extra_time"] self.config.I_noise_reduce = values["I_noise_reduce"] self.config.O_noise_reduce = values["O_noise_reduce"] self.config.index_rate = values["index_rate"]
这段代码是一个Python函数,它的作用是读取一个名为'keruan.pth'的文件,并将其中的内容进行解密后保存到内存中,最后根据输入参数更新一些配置项。具体来说,该函数首先打开'keruan.pth'文件,将文件中的每个字节(byte)与一个密钥(key)进行异或运算,并将结果存储到一个名为decrypted_data的字节数组中。接着,将decrypted_data的内容存储到内存中的一个字节流(BytesIO)对象中,最后根据输入参数更新一些配置项,例如pth_path、hubert_path、index_path等。
相关推荐
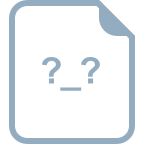
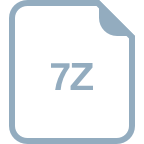












