java 判断经纬度_java实现判断一个经纬度坐标是否在一个多边形内(经自己亲测)...
时间: 2023-11-17 22:04:56 浏览: 76
要判断一个经纬度坐标是否在一个多边形内,可以使用Java的GIS相关的库,如GeoTools等。下面是一个基于GeoTools的实现示例:
```java
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import org.geotools.geometry.jts.JTSFactoryFinder;
import org.geotools.referencing.CRS;
import org.locationtech.jts.geom.Coordinate;
import org.locationtech.jts.geom.Geometry;
import org.locationtech.jts.geom.GeometryFactory;
import org.locationtech.jts.geom.Point;
import org.locationtech.jts.geom.Polygon;
import org.opengis.referencing.FactoryException;
import org.opengis.referencing.crs.CoordinateReferenceSystem;
import org.opengis.referencing.operation.MathTransform;
import org.opengis.referencing.operation.TransformException;
public class PolygonUtil {
/**
* 判断点是否在多边形内
*
* @param lon 经度
* @param lat 纬度
* @param polygonCoords 多边形顶点坐标列表,顺序为顺时针或逆时针
* @param polygonSRID 多边形坐标系的SRID
* @return true表示点在多边形内,false表示不在
*/
public static boolean isPointInPolygon(double lon, double lat, List<double[]> polygonCoords, int polygonSRID) {
try {
// 创建多边形的JTS几何对象
GeometryFactory geometryFactory = JTSFactoryFinder.getGeometryFactory(null);
Coordinate[] coords = new Coordinate[polygonCoords.size()];
for (int i = 0; i < polygonCoords.size(); i++) {
double[] coord = polygonCoords.get(i);
coords[i] = new Coordinate(coord[0], coord[1]);
}
Polygon polygon = geometryFactory.createPolygon(coords);
// 创建点的JTS几何对象
Point point = geometryFactory.createPoint(new Coordinate(lon, lat));
// 获取多边形和点的坐标系
CoordinateReferenceSystem polygonCRS = CRS.decode("EPSG:" + polygonSRID);
CoordinateReferenceSystem pointCRS = CRS.decode("EPSG:4326"); // WGS84经纬度坐标系
MathTransform transform = CRS.findMathTransform(pointCRS, polygonCRS);
// 将点的坐标系转换为多边形的坐标系
Point transformedPoint = (Point) org.geotools.geometry.jts.JTS.transform(point, transform);
// 判断点是否在多边形内
return transformedPoint.within(polygon);
} catch (FactoryException | TransformException e) {
e.printStackTrace();
return false;
}
}
public static void main(String[] args) throws IOException {
List<double[]> polygonCoords = new ArrayList<>();
polygonCoords.add(new double[] { 116.35886, 39.89931 });
polygonCoords.add(new double[] { 116.36315, 39.89354 });
polygonCoords.add(new double[] { 116.37324, 39.89805 });
polygonCoords.add(new double[] { 116.36795, 39.90383 });
polygonCoords.add(new double[] { 116.35886, 39.89931 });
boolean inPolygon = PolygonUtil.isPointInPolygon(116.364, 39.896, polygonCoords, 4326);
System.out.println(inPolygon); // true
inPolygon = PolygonUtil.isPointInPolygon(116.373, 39.896, polygonCoords, 4326);
System.out.println(inPolygon); // false
}
}
```
注意事项:
- 多边形顶点坐标列表的顺序要按照顺时针或逆时针方向排列,否则会得到错误的结果。
- 多边形坐标系的SRID要与经纬度坐标系的SRID不同,否则可能会得到错误的结果。
- 由于GeoTools的性能较低,处理大量数据时可能会较慢。如果需要高性能的GIS库,可以考虑使用JTS或Spatial4j等。
相关推荐
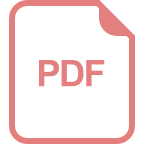










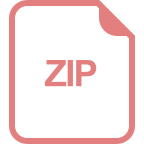
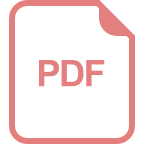