nestjs的module中providers和imports有什么区别,使用起来有什么不一样的
时间: 2024-06-11 16:10:30 浏览: 340
区别:
- `providers` 定义了当前模块中需要注入的服务或者提供者,这些服务或者提供者可以被其他模块或者控制器依赖注入。
- `imports` 定义了当前模块依赖的其他模块,这些模块中定义的服务或者提供者可以被当前模块或者控制器使用。
使用方式不同:
- `providers` 中的服务或者提供者需要使用 `@Injectable()` 装饰器进行注解,同时需要将它们添加到 `providers` 数组中。
- `imports` 中的其他模块需要使用 `@Module()` 装饰器进行注解,同时需要将它们添加到 `imports` 数组中。
在实际使用中,`providers` 和 `imports` 都是用来管理模块中的依赖关系的,但是二者的作用范围不同,`providers` 主要用来管理当前模块中的依赖,`imports` 则主要用来管理当前模块所依赖的其他模块。
相关问题
nestjs rabbitmq 使用
NestJS 是一个用于构建高效、可靠和可扩展的服务器端应用程序的框架。它使用 TypeScript 编写,但也兼容纯 JavaScript。NestJS 提供了对 RabbitMQ 的支持,RabbitMQ 是一个流行的开源消息代理软件,它实现了高级消息队列协议(AMQP)。
在 NestJS 中使用 RabbitMQ 通常会用到第三方库,如 `nestjs-typeorm-rabbitmq` 或者 `amqplib`,这里我们以 `amqplib` 为例说明如何集成 RabbitMQ 到 NestJS 应用中。
1. **安装依赖**:首先需要安装 `amqplib`,可以通过 npm 或 yarn 进行安装。
```bash
npm install amqplib
```
2. **创建 RabbitMQ 服务**:创建一个服务来封装与 RabbitMQ 的交互逻辑。
```typescript
// rabbitmq.service.ts
import * as amqp from 'amqplib';
export class RabbitMQService {
private connection: amqp.Connection;
private channel: amqp.Channel;
async connect(): Promise<void> {
this.connection = await amqp.connect('amqp://localhost');
this.channel = await this.connection.createChannel();
}
async publish(exchange: string, routingKey: string, content: string): Promise<void> {
await this.channel.assertExchange(exchange, 'direct', { durable: false });
this.channel.publish(exchange, routingKey, Buffer.from(content));
}
async close(): Promise<void> {
await this.channel.close();
await this.connection.close();
}
}
```
3. **使用服务**:在需要使用 RabbitMQ 的模块或控制器中注入这个服务,并调用相应的方法。
```typescript
// app.module.ts
import { Module } from '@nestjs/common';
import { RabbitMQService } from './rabbitmq.service';
import { AppController } from './app.controller';
@Module({
imports: [],
controllers: [AppController],
providers: [RabbitMQService],
})
export class AppModule {}
// app.controller.ts
import { Controller, Post } from '@nestjs/common';
import { RabbitMQService } from './rabbitmq.service';
@Controller()
export class AppController {
constructor(private rabbitMQService: RabbitMQService) {}
@Post('send')
async sendMessageToQueue() {
await this.rabbitMQService.connect();
await this.rabbitMQService.publish('exchangeName', 'routingKey', 'Hello, RabbitMQ!');
await this.rabbitMQService.close();
}
}
```
4. **配置和管理连接**:在实际应用中,连接的打开和关闭应该更加谨慎地管理,可能会使用到 NestJS 的生命周期钩子,如 `onModuleInit` 和 `onModuleDestroy`,来管理 RabbitMQ 连接的生命周期。
nestjs 使用cookie
NestJS是一个基于TypeScript的开源Web框架,它在Express之上构建,提供了丰富的功能和模块化设计。如果你想要在NestJS应用中使用cookies进行用户身份验证或存储数据,你可以遵循这些步骤:
1. **安装依赖**:
首先,确保已经安装了`@nestjs/authentication`和`@nestjs/http`模块,它们包含了处理HTTP请求和身份验证的功能。如果还没有安装,可以通过`npm install @nestjs/authentication @nestjs/http`命令添加。
2. **创建服务**:
在`app.module.ts`或相关的模块中,引入`AuthGuard`或自定义的认证策略,并使用`useGlobalGuards`注册它:
```typescript
import { Module, GlobalGuard } from '@nestjs/common';
import { AuthGuard } from '@nestjs/passport';
@Module({
providers: [AuthGuard],
// ...
})
export class AppModule implements GlobalGuard {
canActivate(context: ExecutionContext) {
// 自定义登录检查逻辑
return true;
}
}
```
3. **设置cookie**:
在认证成功后,使用`Response`对象的`setCookie`方法来设置cookie:
```typescript
import { Injectable, CanActivate, HttpStatus, HttpResponse } from '@nestjs/common';
import * as cookieParser from 'cookie-parser';
import * as jwt from 'jsonwebtoken';
@Injectable()
export class JwtAuthGuard extends AuthGuard('jwt') {
canActivate(context: ExecutionContext): boolean {
// ...其他认证逻辑
const user = this.getUserFromToken(); // 获取用户信息
if (!user) {
return false;
}
// 设置cookie
const token = jwt.sign({ userId: user.id }, 'your-secret-key', { expiresIn: '1h' });
const response = context.switchToHttp().getResponse<HttpResponse>();
response.setCookie('jwt', token, { httpOnly: true, maxAge: 60 * 60 * 1000 });
return true;
}
}
```
这里设置了名为`jwt`的cookie,值为JWT令牌,通常`httpOnly`属性会确保cookie不被JavaScript脚本访问,`maxAge`则设置了cookie的有效时间。
4. **验证cookie**:
在每个需要保护的路由或控制器前,使用`@UseGuards(JwtAuthGuard)`装饰器,NestJS会自动在每次请求头中查找`jwt` cookie并解码验证。
阅读全文
相关推荐
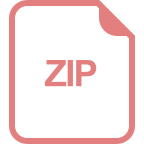
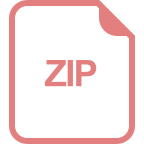
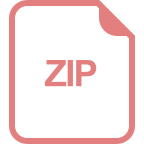
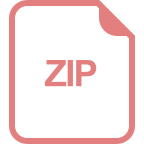
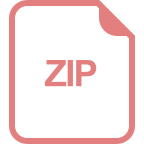
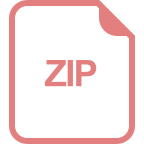
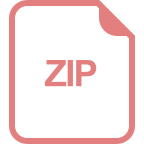
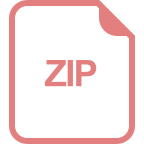
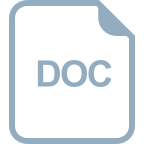
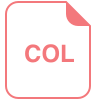
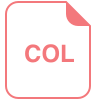
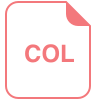
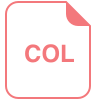



