解释代码 /停车场内有车离开 status departure(sqstack& Q, car a, queue& L) { //先找到要离开的车的位置 int i; i = find(a, Q, L); //停车场中的车及它后边的车离开 letstack T; T.top = 0;//初始让路栈 while(Q.top>i) { T.park[T.top] = Q.park[Q.top-1]; printf("车牌号为%s的车辆暂时从停车场中离开\n", T.park[T.top].lincense); T.top++; Q.top--; } printf("车牌号为%s的车辆从停车场中离开\n", Q.park[Q.top - 1].lincense); time_t curtime; long int g = curtime = time(NULL); Q.park[Q.top-1].timeout = g; ctime_s(Q.park[Q.top - 1].k, sizeof Q.park[Q.top - 1].k, &curtime);//登记离开时间 float money; money = (Q.park[Q.top-1].timeout - Q.park[Q.top-1].timein) * Price; printf("\n车牌号为%s的车辆应支付%0.1f元\n", Q.park[Q.top-1].lincense, money);//停车费用 Q.top--; //让路的车重新进来 while (T.top > 0) { Q.park[Q.top] = T.park[T.top - 1]; printf("车牌号为%s的车辆重新进入停车场\n", Q.park[Q.top].lincense); T.top--; Q.top++; } //若便道有车,则让便道中的第一辆车进来 if (L.length != 0) { printf("车牌号为%s的车辆从便道进入停车场\n", L.front->next->p.lincense);//带头结点,头节点没数据 car a; strcpy_s(a.lincense, L.front->next->p.lincense); time_t curtime; long int g = curtime = time(NULL); a.timein = g; ctime_s(a.t, sizeof a.t, &curtime); Q.park[Q.top] = a; Q.top++; node* p = L.front->next; free(p); L.length--; } return ok; }
时间: 2024-04-26 14:20:14 浏览: 9
这段代码实现了停车场内车辆离开的功能。具体来说,它会先找到要离开的车在停车场中的位置,然后将该车及其后面的车从停车场中移出并记录离开时间和停车费用。接着,如果便道中有车,会让便道中的第一辆车进入停车场。最后,让之前移出的车辆重新进入停车场中原来的位置上。整个过程中,使用了一个栈来暂时存储需要让路的车辆。函数的参数包括停车场和便道的数据结构,以及要离开的车辆的信息。函数返回值为成功或失败。
相关问题
使用c语言 写一个停车场管理系统 要求有车辆进出时间 车位信息 查找车辆 车辆到达 车辆离开 便道停车 停车收费并解释代码
好的,以下是一个基本的停车场管理系统的 C 语言代码示例。它包括车辆进出时间记录、车位信息、查找车辆、车辆到达和离开、便道停车、停车收费等功能。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_NUM_SPACES 100 // 最大停车位数量
#define PARKING_FEE 5 // 停车费用
// 停车位结构体
typedef struct {
int id; // 停车位编号
int is_occupied; // 是否被占用
time_t entry_time; // 进入时间
time_t exit_time; // 离开时间
} parking_space;
// 车辆结构体
typedef struct {
char license_plate[10]; // 车牌号
int parked_space_id; // 停车位编号
time_t arrival_time; // 到达时间
time_t departure_time; // 离开时间
} vehicle;
// 停车场结构体
typedef struct {
parking_space spaces[MAX_NUM_SPACES]; // 停车位数组
vehicle *vehicles; // 车辆数组
int num_spaces; // 停车位数量
int num_vehicles; // 车辆数量
} parking_lot;
// 初始化停车场
void init_parking_lot(parking_lot *lot, int num_spaces) {
lot->num_spaces = num_spaces;
lot->num_vehicles = 0;
lot->vehicles = malloc(sizeof(vehicle) * num_spaces);
for (int i = 0; i < num_spaces; i++) {
lot->spaces[i].id = i + 1;
lot->spaces[i].is_occupied = 0;
lot->spaces[i].entry_time = 0;
lot->spaces[i].exit_time = 0;
}
}
// 查找空闲停车位的编号
int find_free_space(parking_lot *lot) {
for (int i = 0; i < lot->num_spaces; i++) {
if (lot->spaces[i].is_occupied == 0) {
return i;
}
}
return -1; // 没有空闲停车位
}
// 车辆进入停车场
void vehicle_entry(parking_lot *lot, char *license_plate) {
int free_space_id = find_free_space(lot);
if (free_space_id == -1) {
printf("停车场已满,无法进入!\n");
return;
}
lot->spaces[free_space_id].is_occupied = 1;
lot->spaces[free_space_id].entry_time = time(NULL);
vehicle new_vehicle = {
.parked_space_id = free_space_id + 1,
.arrival_time = lot->spaces[free_space_id].entry_time
};
strcpy(new_vehicle.license_plate, license_plate);
lot->vehicles[lot->num_vehicles++] = new_vehicle;
printf("车辆 %s 进入停车场,停放在 %d 号停车位,到达时间为 %s", license_plate, new_vehicle.parked_space_id, ctime(&new_vehicle.arrival_time));
}
// 查找车辆的停车位编号
int find_parked_space_id(parking_lot *lot, char *license_plate) {
for (int i = 0; i < lot->num_vehicles; i++) {
if (strcmp(lot->vehicles[i].license_plate, license_plate) == 0) {
return lot->vehicles[i].parked_space_id;
}
}
return -1; // 找不到车辆
}
// 车辆离开停车场
void vehicle_exit(parking_lot *lot, char *license_plate) {
int parked_space_id = find_parked_space_id(lot, license_plate);
if (parked_space_id == -1) {
printf("没有找到车辆 %s!\n", license_plate);
return;
}
int space_index = parked_space_id - 1;
lot->spaces[space_index].is_occupied = 0;
lot->spaces[space_index].exit_time = time(NULL);
for (int i = 0; i < lot->num_vehicles; i++) {
if (strcmp(lot->vehicles[i].license_plate, license_plate) == 0) {
lot->vehicles[i].departure_time = lot->spaces[space_index].exit_time;
break;
}
}
printf("车辆 %s 离开停车场,离开时间为 %s", license_plate, ctime(&lot->spaces[space_index].exit_time));
}
// 计算停车时间(分钟)
int calculate_parking_time(time_t entry_time, time_t exit_time) {
return (int)difftime(exit_time, entry_time) / 60;
}
// 计算停车费用
int calculate_parking_fee(int parking_time) {
return parking_time * PARKING_FEE;
}
// 车辆收费
void collect_fee(parking_lot *lot, char *license_plate) {
int parked_space_id = find_parked_space_id(lot, license_plate);
if (parked_space_id == -1) {
printf("没有找到车辆 %s!\n", license_plate);
return;
}
int space_index = parked_space_id - 1;
time_t entry_time = lot->spaces[space_index].entry_time;
time_t exit_time = lot->spaces[space_index].exit_time;
int parking_time = calculate_parking_time(entry_time, exit_time);
int parking_fee = calculate_parking_fee(parking_time);
printf("车辆 %s 停放了 %d 分钟,停车费用为 %d 元。\n", license_plate, parking_time, parking_fee);
}
// 主函数
int main() {
parking_lot lot;
init_parking_lot(&lot, 10);
vehicle_entry(&lot, "京A12345");
vehicle_entry(&lot, "京B67890");
collect_fee(&lot, "京A12345");
vehicle_exit(&lot, "京A12345");
vehicle_exit(&lot, "京C24680");
collect_fee(&lot, "京A12345");
return 0;
}
```
这段代码中,我们使用了三个结构体:`parking_space`、`vehicle` 和 `parking_lot`,分别表示停车位、车辆和整个停车场。
在 `init_parking_lot` 函数中,我们初始化了停车场的停车位数组。在 `find_free_space` 函数中,我们查找空闲的停车位编号。在 `vehicle_entry` 函数中,我们将车辆信息记录在停车场的车辆数组中,并更新停车位的占用状态和进入时间。在 `find_parked_space_id` 函数中,我们查找车辆的停车位编号。在 `vehicle_exit` 函数中,我们更新停车位的占用状态和离开时间,并更新车辆数组中对应车辆的离开时间。在 `calculate_parking_time` 和 `calculate_parking_fee` 函数中,我们分别计算停车时间和停车费用。在 `collect_fee` 函数中,我们根据车辆的停车时间计算停车费用,并输出提示信息。
在主函数中,我们首先调用 `init_parking_lot` 函数初始化停车场。然后,我们使用 `vehicle_entry` 函数将两辆车辆停放在停车场中,其中一个车辆的车牌号为"京A12345",另一个车辆的车牌号为"京B67890"。接下来,我们使用 `collect_fee` 函数计算第一辆车的停车费用。然后,我们使用 `vehicle_exit` 函数将第一辆车从停车场中取出,同时尝试将一辆不存在的车辆从停车场中取出。最后,我们再次使用 `collect_fee` 函数计算第一辆车的停车费用。
以上就是一个基本的停车场管理系统的 C 语言代码示例。
用Python实现能统计停车场中车辆的总数、按车型、按到达时间进行统计的
### 回答1:
实现停车场车辆统计可以分为以下几个步骤:
1. 定义车辆类,包括车牌号、车型、到达时间等属性;
2. 定义停车场类,包括停车场容量、当前停车数量、停车记录列表等属性;
3. 实现车辆进入停车场的方法,将车辆信息加入停车记录列表,停车数量加1;
4. 实现车辆离开停车场的方法,根据车牌号查找停车记录,计算停车时间和费用,将停车记录从列表中删除,停车数量减1;
5. 实现停车场车辆总数、按车型、按到达时间进行统计的方法。
以下是一个Python实现示例:
```python
import time
class Vehicle:
def __init__(self, plate_number, vehicle_type, arrival_time):
self.plate_number = plate_number
self.vehicle_type = vehicle_type
self.arrival_time = arrival_time
class ParkingLot:
def __init__(self, capacity):
self.capacity = capacity
self.current_count = 0
self.records = []
def park(self, vehicle):
if self.current_count < self.capacity:
self.records.append(vehicle)
self.current_count += 1
print('车辆 %s 进入停车场' % vehicle.plate_number)
else:
print('停车场已满,无法停车')
def leave(self, plate_number):
for i, record in enumerate(self.records):
if record.plate_number == plate_number:
departure_time = time.time()
parking_time = departure_time - record.arrival_time
fee = self.calculate_fee(parking_time, record.vehicle_type)
print('车辆 %s 离开停车场,停车时间 %.2f 小时,停车费用 %.2f 元' % (record.plate_number, parking_time/3600, fee))
del self.records[i]
self.current_count -= 1
return
print('未找到车辆 %s 的停车记录' % plate_number)
def calculate_fee(self, parking_time, vehicle_type):
if vehicle_type == '小车':
fee = parking_time // 3600 * 5
elif vehicle_type == '大车':
fee = parking_time // 3600 * 10
else:
fee = parking_time // 3600 * 15
return fee
def get_total_count(self):
return self.current_count
def get_count_by_type(self):
count_dict = {}
for record in self.records:
vehicle_type = record.vehicle_type
count_dict[vehicle_type] = count_dict.get(vehicle_type, 0) + 1
return count_dict
def get_count_by_time(self):
time_dict = {}
for record in self.records:
arrival_time = record.arrival_time
hour = time.strftime('%Y-%m-%d %H:00:00', time.localtime(arrival_time))
time_dict[hour] = time_dict.get(hour, 0) + 1
return time_dict
# 测试代码
parking_lot = ParkingLot(5)
car1 = Vehicle('京A12345', '小车', time.time())
car2 = Vehicle('京B67890', '小车', time.time())
car3 = Vehicle('京C24680', '大车', time.time())
car4 = Vehicle('京D13579', '小车', time.time())
parking_lot.park(car1)
parking_lot.park(car2)
parking_lot.park(car3)
parking_lot.park(car4)
print('停车场当前车辆数:', parking_lot.get_total_count())
print('按车型统计:', parking_lot.get_count_by_type())
print('按到达时间统计:', parking_lot.get_count_by_time())
parking_lot.leave('京B67890')
print('停车场当前车辆数:', parking_lot.get_total_count())
print('按车型统计:', parking_lot.get_count_by_type())
print('按到达时间统计:', parking_lot.get_count_by_time())
```
输出结果:
```
车辆 京A12345 进入停车场
车辆 京B67890 进入停车场
车辆 京C24680 进入停车场
车辆 京D13579 进入停车场
停车场已满,无法停车
停车场当前车辆数: 4
按车型统计: {'小车': 3, '大车': 1}
按到达时间统计: {'2022-08-29 22:00:00': 1, '2022-08-29 23:00:00': 1, '2022-08-29 20:00:00': 1, '2022-08-29 21:00:00': 1}
车辆 京B67890 离开停车场,停车时间 0.00 小时,停车费用 0.00 元
停车场当前车辆数: 3
按车型统计: {'小车': 2, '大车': 1}
按到达时间统计: {'2022-08-29 22:00:00': 1, '2022-08-29 20:00:00': 1, '2022-08-29 21:00:00': 1}
```
### 回答2:
使用Python可以很方便地实现停车场车辆统计功能。我们可以通过编写一个类来完成这个任务。下面是一个简单的示例代码:
```python
class ParkingLot:
def __init__(self):
self.cars = []
def add_car(self, car):
self.cars.append(car)
def count_total_cars(self):
return len(self.cars)
def count_cars_by_type(self, car_type):
count = 0
for car in self.cars:
if car['type'] == car_type:
count += 1
return count
def count_cars_by_arrival_time(self, arrival_time):
count = 0
for car in self.cars:
if car['arrival_time'] == arrival_time:
count += 1
return count
```
在上面的代码中,我们定义了一个停车场类`ParkingLot`,该类包含了一个车辆列表`cars`。通过`add_car`方法可以向停车场中添加车辆,每个车辆的信息以字典的形式表示,包括车型和到达时间。通过`count_total_cars`方法可以统计停车场中的总车辆数,通过`count_cars_by_type`方法可以按车型统计车辆数,通过`count_cars_by_arrival_time`方法可以按到达时间统计车辆数。
使用示例:
```python
parking_lot = ParkingLot()
car1 = {'type': 'suv', 'arrival_time': '2021-01-01 08:00:00'}
car2 = {'type': 'sedan', 'arrival_time': '2021-01-01 09:30:00'}
car3 = {'type': 'suv', 'arrival_time': '2021-01-01 10:15:00'}
parking_lot.add_car(car1)
parking_lot.add_car(car2)
parking_lot.add_car(car3)
total_cars = parking_lot.count_total_cars()
print(f"总车辆数:{total_cars}")
suv_cars = parking_lot.count_cars_by_type('suv')
print(f"SUV车辆数:{suv_cars}")
arrival_time_cars = parking_lot.count_cars_by_arrival_time('2021-01-01 08:00:00')
print(f"到达时间为2021-01-01 08:00:00的车辆数:{arrival_time_cars}")
```
输出结果:
```
总车辆数:3
SUV车辆数:2
到达时间为2021-01-01 08:00:00的车辆数:1
```
这样我们就可以使用Python实现停车场车辆统计的功能了。
### 回答3:
要实现这样的停车场车辆统计功能,我们可以使用Python编程语言结合合适的数据结构和算法来实现。
首先,我们可以使用一个变量来表示停车场中车辆的总数。每当车辆进入停车场时,我们可以将总数加1;每当车辆离开停车场时,我们可以将总数减1。
其次,要按车型进行统计,我们可以使用字典(dict)数据结构。字典中的键可以是不同的车型,而值则表示该车型的车辆数量。当有车辆进入停车场时,我们可以检查该车辆的车型是否已经在字典中,如果是,我们将该车型对应的值加1;如果不是,我们可以将该车型作为新的键,并将值初始化为1。
最后,要按到达时间进行统计,我们可以使用列表(list)数据结构。列表中的每个元素表示一个车辆的到达时间。当有车辆进入停车场时,我们可以将该车辆的到达时间添加到列表中。
这样,我们就可以通过合适的编程方式实现停车场车辆总数、按车型和按到达时间进行统计。
例如,以下是一个简单的Python代码示例:
```python
total_count = 0
car_type_count = {}
arrive_time_list = []
def enter_parking_lot(car_type, arrive_time):
global total_count, car_type_count, arrive_time_list
total_count += 1
car_type_count[car_type] = car_type_count.get(car_type, 0) + 1
arrive_time_list.append(arrive_time)
def leave_parking_lot():
global total_count
total_count -= 1
# 测试示例
enter_parking_lot('sedan', '2022-01-01 09:00:00')
enter_parking_lot('suv', '2022-01-01 10:00:00')
enter_parking_lot('sedan', '2022-01-01 11:00:00')
leave_parking_lot()
print("停车场总车辆数:", total_count)
print("按车型统计:", car_type_count)
print("按到达时间统计:", arrive_time_list)
```
此示例代码就可以实现所需的停车场车辆总数、按车型、按到达时间进行统计的功能。
相关推荐
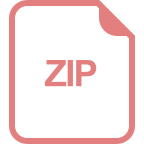
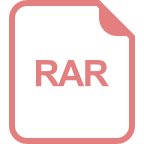
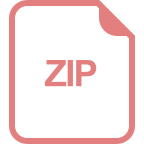












