2)利用lseek空洞文件原理,完成对文件的分片拷贝
时间: 2024-04-12 15:32:13 浏览: 11
可以利用lseek函数的空洞文件原理来实现对文件的分片拷贝。空洞文件是指文件中存在一些数据块没有被实际占用,这些数据块被称为空洞。lseek函数可以用来改变文件的读写位置,并且当设置的位置超过文件的大小时,文件会被自动扩展,并在扩展的空间中填充0。
下面是一个使用lseek函数进行分片拷贝的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
void copyFile(char* srcPath, char* destPath, off_t start, off_t size) {
int srcFd = open(srcPath, O_RDONLY);
int destFd = open(destPath, O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (srcFd == -1 || destFd == -1) {
perror("Failed to open file");
exit(1);
}
off_t fileSize = lseek(srcFd, 0, SEEK_END);
lseek(srcFd, start, SEEK_SET);
char buffer[1024];
off_t remaining = size;
while (remaining > 0) {
ssize_t bytesRead = read(srcFd, buffer, sizeof(buffer));
if (bytesRead > remaining) {
bytesRead = remaining;
}
ssize_t bytesWritten = write(destFd, buffer, bytesRead);
if (bytesWritten != bytesRead) {
perror("Failed to write file");
exit(1);
}
remaining -= bytesRead;
}
close(srcFd);
close(destFd);
}
int main() {
char* srcPath = "source_file.txt";
char* destPath = "destination_file.txt";
off_t start = 100; // 起始位置
off_t size = 200; // 拷贝的大小
copyFile(srcPath, destPath, start, size);
return 0;
}
```
上述代码中,copyFile函数用于实现文件的分片拷贝。它首先打开源文件和目标文件,然后使用lseek函数将源文件的读写位置移动到指定的起始位置start。接下来,使用read函数从源文件中读取数据到缓冲区buffer中,然后使用write函数将缓冲区中的数据写入目标文件。这个过程会循环执行,直到拷贝指定大小的数据或者源文件的数据读取完毕。最后,关闭源文件和目标文件的文件描述符。
你可以根据实际需求修改代码中的文件路径、起始位置和拷贝大小等参数。注意,这里的起始位置和拷贝大小都是以字节为单位的偏移量。
相关推荐
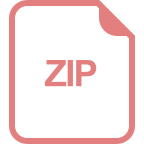














