改造Set类,重载+(并集)、(-)差集、*(交集)、<<(输出)、>>(输入)和函数调用操作符(添加一个元素)
时间: 2023-10-17 22:05:08 浏览: 37
以下是改造后的Set类代码:
```cpp
#include <iostream>
#include <set>
using namespace std;
class Set {
private:
set<int> s;
public:
Set() {}
// 添加一个元素
void operator()(int x) {
s.insert(x);
}
// 并集
Set operator+(const Set& other) const {
Set res;
set_union(s.begin(), s.end(), other.s.begin(), other.s.end(), inserter(res.s, res.s.begin()));
return res;
}
// 差集
Set operator-(const Set& other) const {
Set res;
set_difference(s.begin(), s.end(), other.s.begin(), other.s.end(), inserter(res.s, res.s.begin()));
return res;
}
// 交集
Set operator*(const Set& other) const {
Set res;
set_intersection(s.begin(), s.end(), other.s.begin(), other.s.end(), inserter(res.s, res.s.begin()));
return res;
}
// 输出
friend ostream& operator<<(ostream& os, const Set& obj) {
os << "{";
for (auto it = obj.s.begin(); it != obj.s.end(); ++it) {
os << *it;
if (next(it) != obj.s.end())
os << ", ";
}
os << "}";
return os;
}
// 输入
friend istream& operator>>(istream& is, Set& obj) {
int n;
is >> n;
for (int i = 0; i < n; ++i) {
int x;
is >> x;
obj.s.insert(x);
}
return is;
}
};
```
使用示例:
```cpp
int main() {
Set s1, s2;
cout << "Enter set 1: ";
cin >> s1;
cout << "Enter set 2: ";
cin >> s2;
Set s3 = s1 + s2;
Set s4 = s1 - s2;
Set s5 = s1 * s2;
cout << "Set 1: " << s1 << endl;
cout << "Set 2: " << s2 << endl;
cout << "Union: " << s3 << endl;
cout << "Difference: " << s4 << endl;
cout << "Intersection: " << s5 << endl;
s1(10);
cout << "After adding 10 to set 1: " << s1 << endl;
return 0;
}
```
相关推荐
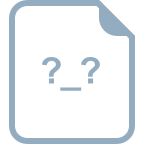
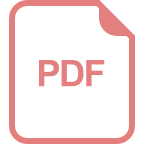
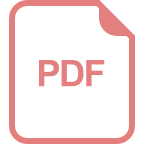













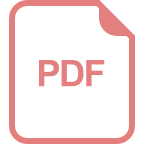
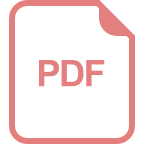