rclcpp::Time when = this->get_clock()->now() - rclcpp::Duration(5, 0); t = tf_buffer_->lookupTransfo...
时间: 2023-06-25 09:04:05 浏览: 111
这段代码是使用ROS 2中的rclcpp库实现的,目的是获取一个时间点5秒前某个坐标系的变换关系。具体来说:
- `this->get_clock()->now()` 获取当前时间点;
- `rclcpp::Duration(5, 0)` 创建一个时间间隔,表示5秒;
- `this->get_clock()->now() - rclcpp::Duration(5, 0)` 计算5秒前的时间点;
- `tf_buffer_->lookupTransform(target_frame, source_frame, when)` 查询在指定时间点`when`时,从`source_frame`到`target_frame`的坐标系变换关系。
其中,`tf_buffer_`是一个保存了所有坐标系变换关系的缓存,可以通过`lookupTransform()`函数查询两个坐标系之间的变换关系。
相关问题
c++ string "%02d:%02d:%02d:%06ld", timenow->tm_hour, timenow->tm_min, timenow->tm_sec, time.tv_usec
This is a C string that uses format specifiers to construct a message that includes the current time. The format specifiers are:
- `%d`: integer decimal format
- `%ld`: long integer decimal format
The message format is:
`"\x02d:\x02d:\x02d:\x06ld"`
- `\x02`: represents the ASCII control character STX (start of text), which is equivalent to character 2.
- `\x06`: represents the ASCII control character ACK (acknowledge), which is equivalent to character 6.
When the string is printed, the format specifiers will be replaced with the corresponding values provided as arguments. `timenow->tm_hour`, `timenow->tm_min`, and `timenow->tm_sec` are integers representing the current hour, minute, and second, respectively. `time.tv_usec` is a long integer representing the current microsecond.
For example, if the current time is 01:23:45.678910, the string would be printed as:
`"\x021:\x023:\x024:\x06678910"`
picture->pkt_dts = p->avpkt->dts;
This line of code sets the `pkt_dts` field of a `picture` struct to the `dts` field of the `avpkt` struct of another struct `p`.
`pkt_dts` represents the Decoding Time Stamp of a video frame, which is the timestamp indicating when the frame should be decoded and displayed. `avpkt` is an `AVPacket` struct in the FFmpeg library, which contains compressed data of a video or audio frame. `dts` stands for Decoding Time Stamp and is a field in the `AVPacket` struct that holds the decoding timestamp of the packet.
Therefore, this line of code is used to assign the decoding timestamp of a video frame to the `pkt_dts` field of a `picture` struct, which can be used in further processing of the video frames.
相关推荐
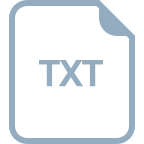
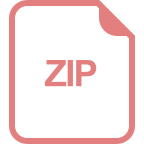













