首先,定义一个矩阵类作为基类,类中需要包含维度值m(行)和n(列)。并在构造函数中动态申请内存空间,实例化m*n的int型二维数组,重载+和-作为矩阵加减法运算符,包含按照m行n列打印出矩阵的show方法,析构函数包含动态释放内存空间。 接下来,定义一个4*4方阵类作为矩阵类的派生类,包含成员方法求行列式的值,包含成员方法左乘矩阵打印出结果,注意规格检查。 最后,定义一个主函数,随机生成5*6矩阵A,5*6矩阵B,5*6矩阵C,计算A+B,A-C;随机生成4*4方阵D,4*4方阵E,计算D*E,计算D和E的行列式,判断D*A的可计算性。
时间: 2023-10-14 07:06:01 浏览: 68
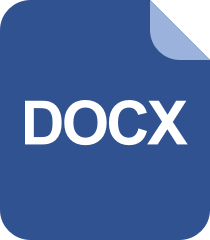
派生类构造函数和析构函数的执行顺序
以下是代码实现:
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
class Matrix {
public:
Matrix(int row, int col) : m(row), n(col) {
data = new int*[m];
for (int i = 0; i < m; i++) {
data[i] = new int[n];
}
}
virtual ~Matrix() {
for (int i = 0; i < m; i++) {
delete[] data[i];
}
delete[] data;
}
Matrix operator+(const Matrix& other) const {
Matrix res(m, n);
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
res.data[i][j] = data[i][j] + other.data[i][j];
}
}
return res;
}
Matrix operator-(const Matrix& other) const {
Matrix res(m, n);
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
res.data[i][j] = data[i][j] - other.data[i][j];
}
}
return res;
}
void show() const {
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
cout << data[i][j] << " ";
}
cout << endl;
}
}
protected:
int m;
int n;
int** data;
};
class SquareMatrix : public Matrix {
public:
SquareMatrix() : Matrix(4, 4) {}
int det() const {
if (m != n) {
cerr << "Not a square matrix!" << endl;
return 0;
}
if (m == 1) {
return data[0][0];
}
int res = 0;
for (int i = 0; i < m; i++) {
SquareMatrix tmp(m - 1, m - 1);
for (int j = 1; j < m; j++) {
for (int k = 0; k < m; k++) {
if (k < i) {
tmp.data[j - 1][k] = data[j][k];
} else if (k > i) {
tmp.data[j - 1][k - 1] = data[j][k];
}
}
}
res += data[0][i] * (i % 2 == 0 ? 1 : -1) * tmp.det();
}
return res;
}
void leftMultiply(const Matrix& other) const {
if (n != other.m) {
cerr << "Invalid matrix size for multiplication!" << endl;
return;
}
SquareMatrix tmp(4, 4);
for (int i = 0; i < m; i++) {
for (int j = 0; j < other.n; j++) {
tmp.data[i][j] = 0;
for (int k = 0; k < n; k++) {
tmp.data[i][j] += data[i][k] * other.data[k][j];
}
}
}
tmp.show();
}
};
int main() {
srand(time(nullptr));
Matrix A(5, 6), B(5, 6), C(5, 6);
for (int i = 0; i < A.m; i++) {
for (int j = 0; j < A.n; j++) {
A.data[i][j] = rand() % 10;
B.data[i][j] = rand() % 10;
}
}
cout << "Matrix A:" << endl;
A.show();
cout << "Matrix B:" << endl;
B.show();
C = A + B;
cout << "A + B:" << endl;
C.show();
C = A - B;
cout << "A - B:" << endl;
C.show();
SquareMatrix D, E;
for (int i = 0; i < D.m; i++) {
for (int j = 0; j < D.n; j++) {
D.data[i][j] = rand() % 10;
E.data[i][j] = rand() % 10;
}
}
cout << "Matrix D:" << endl;
D.show();
cout << "Matrix E:" << endl;
E.show();
cout << "D * E:" << endl;
D.leftMultiply(E);
cout << "det(D): " << D.det() << endl;
cout << "det(E): " << E.det() << endl;
cout << "D * A:" << endl;
D.leftMultiply(A);
return 0;
}
```
以上代码中,Matrix类是矩阵类的基类,实现了矩阵的加减法和打印方法。SquareMatrix类是矩阵类的派生类,继承了Matrix类的成员和方法,并实现了求行列式和左乘矩阵的方法。在主函数中,随机生成两个5*6矩阵A和B,计算A+B和A-B;随机生成两个4*4方阵D和E,计算D*E,计算D和E的行列式,判断D*A的可计算性。
阅读全文
相关推荐
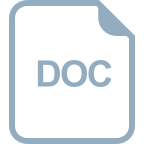
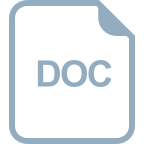



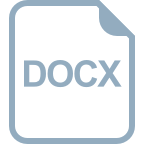
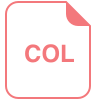
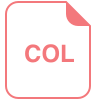
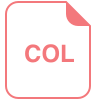
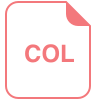
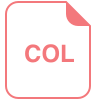
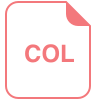
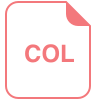
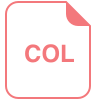
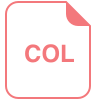
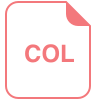

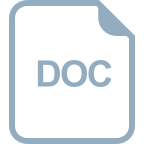