int WINAPI WinMain
时间: 2023-11-22 18:52:04 浏览: 45
`WinMain` 是 Windows 程序的入口函数,它是一个特殊的函数,由编译器调用。它的原型如下:
```c++
int WINAPI WinMain(
HINSTANCE hInstance,
HINSTANCE hPrevInstance,
LPSTR lpCmdLine,
int nShowCmd
);
```
参数说明:
- `hInstance`:当前应用程序实例的句柄。
- `hPrevInstance`:先前运行的应用程序实例的句柄,已经废弃。
- `lpCmdLine`:命令行参数,用于传递给当前应用程序的命令行参数。
- `nShowCmd`:指定应用程序窗口的初始显示状态(最小化、最大化或正常大小)。
`WinMain` 函数的返回值是整数类型,表示应用程序的退出状态。当函数返回时,进程终止并且返回该值。
相关问题
int winapi winmain
winapi是Windows应用程序编程接口的缩写,是一组用于开发Windows操作系统应用程序的函数、数据结构和常量的集合。
winmain是Windows应用程序的入口函数,它是由WinMain或wWinMain调用的。在winmain中,程序可以进行初始化、创建窗口、处理消息等操作。
const TCHAR szOperation[] = _T("open"); const TCHAR szAddress[] = _T("http://www.ssoier.cn:8088/ocr/index.php"); int WINAPI WinMain(HINSTANCE hInst, HINSTANCE, LPSTR lpCmd, int nShow); { HINSTANCE hRslt = ShellExecute(NULL, szOperation, szAddress, NULL, NULL, SW_SHOWNORMAL); assert(hRslt > (HINSTANCE)HINSTANCE_ERROR); exit(100); }
这段代码是一个简单的C++程序,它使用了Windows API函数`ShellExecute`来打开一个指定网址的默认浏览器。在`WinMain`函数中,我们调用`ShellExecute`函数,并传递了打开操作、网址以及其他参数。然后使用`assert`断言来检查函数调用是否成功,最后使用`exit`函数退出程序。
需要注意的是,这段代码并不与题目要求的数学表达式输入内容相关。如果你想要实现自动输入数学表达式并计算结果,可以使用C++的输入输出流和适当的算法来解析和计算表达式。以下是一个示例代码来实现这个功能:
```cpp
#include <iostream>
#include <string>
#include <stack>
using namespace std;
int calculateExpression(const string& expression) {
stack<int> numbers;
stack<char> operators;
for (int i = 0; i < expression.length(); i++) {
if (expression[i] >= '0' && expression[i] <= '9') {
int num = 0;
while (i < expression.length() && expression[i] >= '0' && expression[i] <= '9') {
num = num * 10 + (expression[i] - '0');
i++;
}
i--;
numbers.push(num);
}
else if (expression[i] == '+' || expression[i] == '-') {
while (!operators.empty() && (operators.top() == '+' || operators.top() == '-' ||
operators.top() == '*' || operators.top() == '/')) {
int b = numbers.top();
numbers.pop();
int a = numbers.top();
numbers.pop();
char op = operators.top();
operators.pop();
if (op == '+') {
numbers.push(a + b);
}
else if (op == '-') {
numbers.push(a - b);
}
else if (op == '*') {
numbers.push(a * b);
}
else if (op == '/') {
numbers.push(a / b);
}
}
operators.push(expression[i]);
}
else if (expression[i] == '*' || expression[i] == '/') {
while (!operators.empty() && (operators.top() == '*' || operators.top() == '/')) {
int b = numbers.top();
numbers.pop();
int a = numbers.top();
numbers.pop();
char op = operators.top();
operators.pop();
if (op == '*') {
numbers.push(a * b);
}
else if (op == '/') {
numbers.push(a / b);
}
}
operators.push(expression[i]);
}
}
while (!operators.empty()) {
int b = numbers.top();
numbers.pop();
int a = numbers.top();
numbers.pop();
char op = operators.top();
operators.pop();
if (op == '+') {
numbers.push(a + b);
}
else if (op == '-') {
numbers.push(a - b);
}
else if (op == '*') {
numbers.push(a * b);
}
else if (op == '/') {
numbers.push(a / b);
}
}
return numbers.top();
}
int main() {
string expression;
cout << "请输入数学表达式: ";
cin >> expression;
int result = calculateExpression(expression);
cout << "结果为: " << result << endl;
return 0;
}
```
这个示例代码会提示用户输入一个数学表达式,然后使用`calculateExpression`函数计算表达式的结果,并输出结果。你可以根据你的具体需求进行修改和扩展。
相关推荐
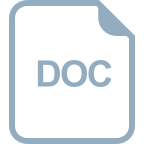
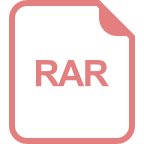
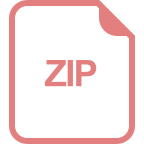











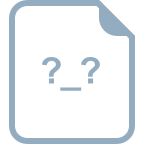
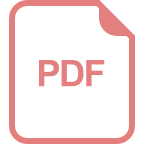