将此段代码翻译为C#代码:#include <windows.h> #include <stdio.h> #include <stdlib.h> #include <math.h> FILE *fp; bool getPixelBool(int x, int y){ int *numa = new int[y * 2]; int *numb = new int[y * 2]; numa[0] = 1; for(int b = 0; b < y * 2; b++){ for(int a = 0; a < b; a++){ if(a == 0){ numb[a] = 1; }else{ numb[a] = numa[a - 1] + numa[a]; } } for(int i = 0; i < b; i++){ numa[i] = numb[i]; } } if(numa[x] % 2 == 0){ return false; }else{ return true; } } void pixel_write (int r, int g, int b){ static unsigned char color[3]; color[0] = r; color[1] = g; color[2] = b; fwrite (color, 1, 3, fp); } int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpcmdLine, int nShowCmd){ int bgR = 0, bgG = 0, bgB = 0; //背景色 int R = 255, G = 255, B = 255; //前景色 int side = 9; //叠加层数 int picw = ((int)pow(2, side + 1)) - 1, pich = (int)pow(2, side); fp = fopen ("SierpinskiCarpet.ppm","wb"); fprintf (fp, "P6\n%d %d\n255\n", picw, pich); for(int j = 1; j <= pich; j++){ for(int i = 1; i <= picw; i++){ bool colors = false; if(i < (((picw - 1) / 2) - j) || i >= (picw - (((picw - 1) / 2) - j))){ colors = true; }else{ int x = i - (((picw - 1) / 2) - j), y = j; colors = getPixelBool(x, y); } if(colors == false){ pixel_write(bgR, bgG, bgB); }else{ pixel_write(R, G, B); } } } fclose (fp); return 0; }
时间: 2024-01-05 09:04:46 浏览: 33
以下是将该段C++代码翻译为C#代码的示例:
```csharp
using System;
using System.IO;
class Program
{
static FileStream fp;
static bool GetPixelBool(int x, int y)
{
int[] numa = new int[y * 2];
int[] numb = new int[y * 2];
numa[0] = 1;
for (int b = 0; b < y * 2; b++)
{
for (int a = 0; a < b; a++)
{
if (a == 0)
{
numb[a] = 1;
}
else
{
numb[a] = numa[a - 1] + numa[a];
}
}
for (int i = 0; i < b; i++)
{
numa[i] = numb[i];
}
}
if (numa[x] % 2 == 0)
{
return false;
}
else
{
return true;
}
}
static void PixelWrite(byte r, byte g, byte b)
{
byte[] color = new byte[] { r, g, b };
fp.Write(color, 0, 3);
}
static void Main()
{
int bgR = 0, bgG = 0, bgB = 0; //背景色
int R = 255, G = 255, B = 255; //前景色
int side = 9; //叠加层数
int picw = (int)Math.Pow(2, side + 1) - 1, pich = (int)Math.Pow(2, side);
fp = new FileStream("SierpinskiCarpet.ppm", FileMode.Create);
StreamWriter sw = new StreamWriter(fp);
sw.Write("P6\n{0} {1}\n255\n", picw, pich);
sw.Flush();
for (int j = 1; j <= pich; j++)
{
for (int i = 1; i <= picw; i++)
{
bool colors = false;
if (i < (((picw - 1) / 2) - j) || i >= (picw - (((picw - 1) / 2) - j))))
{
colors = true;
}
else
{
int x = i - (((picw - 1) / 2) - j), y = j;
colors = GetPixelBool(x, y);
}
if (colors == false)
{
PixelWrite((byte)bgR, (byte)bgG, (byte)bgB);
}
else
{
PixelWrite((byte)R, (byte)G, (byte)B);
}
}
}
fp.Close();
}
}
```
在C#中,我们使用`FileStream`来创建文件和写入数据,使用`StreamWriter`来写入文件头等信息。C++中的`FILE`结构体在C#中没有对应,需要使用`FileStream`来代替。C#中的数组定义方式与C++中不同,需要注意。此外,C++中的`int`类型在C#中对应为`byte`类型,需要进行类型转换。
相关推荐
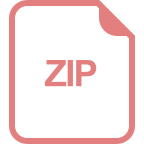
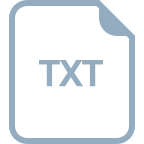
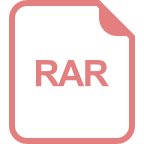














