python让图形旋转
时间: 2023-09-18 18:05:53 浏览: 237
### 回答1:
要让图形旋转,可以使用Python中的turtle模块。turtle模块提供了一个旋转函数`turtle.right(angle)`,可以让绘制的图形向右旋转一定的角度。例如,以下代码可以画一个正方形,并让它向右旋转45度:
```python
import turtle
# 画一个正方形
for i in range(4):
turtle.forward(100)
turtle.right(90)
# 向右旋转45度
turtle.right(45)
turtle.done()
```
如果要让图形持续旋转,可以使用循环不断调用旋转函数。例如,以下代码可以不断让一个正方形旋转:
```python
import turtle
# 画一个正方形
for i in range(4):
turtle.forward(100)
turtle.right(90)
# 不断旋转
while True:
turtle.right(1)
turtle.done()
```
这里的`while True`表示无限循环,`turtle.right(1)`表示每次向右旋转1度。你可以根据需要调整旋转的角度和速度。
### 回答2:
Python可以使用不同的库来实现图形的旋转,其中比较常用的是turtle库和matplotlib库。
在turtle库中,我们可以使用turtle.rotate(angle)函数来实现图形的旋转。这个函数可以让海龟(turtle)对象按照指定的角度angle旋转,正值表示顺时针旋转,负值表示逆时针旋转。
例如,下面的代码使用turtle库创建一个正方形,并将其旋转45度:
import turtle
# 创建一个海龟对象
t = turtle.Turtle()
# 绘制一个正方形
for _ in range(4):
t.forward(100)
t.right(90)
# 旋转45度
t.right(45)
turtle.done()
在matplotlib库中,我们可以使用matplotlib.pyplot模块的pyplot.imshow()函数来显示图像,并利用numpy库的rotate()函数来旋转图像。
下面的代码展示了如何使用matplotlib库将一张图像旋转90度,并显示旋转后的图像:
import matplotlib.pyplot as plt
import numpy as np
# 加载图像
image = plt.imread('image.jpg')
# 旋转图像
rotated_image = np.rot90(image)
# 显示旋转后的图像
plt.imshow(rotated_image)
plt.show()
无论是使用turtle库还是matplotlib库,我们都可以通过改变旋转的角度来实现不同程度的旋转,从而使图形在界面上呈现不同的角度。
### 回答3:
Python提供了多种库和工具,可以让图形旋转。其中较为常用的有`pygame`和`turtle`库。
`pygame`是一个开源的Python模块,专门用于多媒体应用程序开发,包括游戏。通过`pygame`库,可以创建窗口和显示图像,并可以使用其内置的变换函数实现图形旋转。下面是一个使用`pygame`库让图形旋转的示例代码:
```python
import pygame
import sys
from pygame.locals import *
# 初始化pygame库
pygame.init()
# 设置窗口尺寸
window_width, window_height = 400, 300
window_surface = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption('旋转图形')
# 加载图像
image_path = 'image.jpg'
image = pygame.image.load(image_path)
image_rect = image.get_rect(center=(window_width/2, window_height/2))
# 设置旋转角度
angle = 0
while True:
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
window_surface.fill((255, 255, 255)) # 清屏
# 绘制旋转后的图像
rotated_image = pygame.transform.rotate(image, angle)
rotated_rect = rotated_image.get_rect(center=image_rect.center)
window_surface.blit(rotated_image, rotated_rect)
# 更新角度
angle += 1
pygame.display.update()
```
`turtle`库是Python的一个图形库,专门用于绘制图形和动画。通过`turtle`库,可以创建一个窗口并在其中绘制图形,然后通过控制海龟的方向和角度来实现图形的旋转。下面是一个使用`turtle`库让图形旋转的示例代码:
```python
import turtle
# 创建画布和画笔
window = turtle.Screen()
pen = turtle.Turtle()
# 绘制图形
def draw_shape():
pen.forward(100)
pen.left(90)
pen.forward(100)
pen.left(90)
pen.forward(100)
pen.left(90)
pen.forward(100)
pen.left(90)
# 设置旋转角度
angle = 0
while True:
window.clear() # 清屏
# 绘制图形
pen.goto(0, 0) # 移动到中心位置
pen.setheading(angle) # 设置海龟的朝向
draw_shape()
# 更新角度
angle += 1
window.update()
```
以上是使用`pygame`和`turtle`库实现图形旋转的示例代码。可以根据实际需求,使用这些库中的函数和方法来实现更加复杂的图形旋转效果。
相关推荐
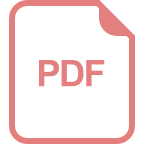
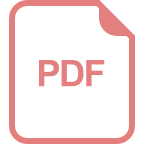
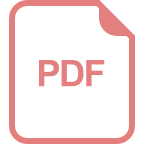
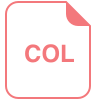
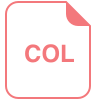
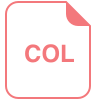
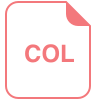
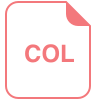









