public int deletestudent(Connection con ,String delid) throws Exception{ String sql = "delete from student where id in("+delid+")"; PreparedStatement p = con.prepareStatement(sql); return p.executeUpdate(); }
时间: 2023-06-19 08:03:58 浏览: 47
这段代码是一个在数据库中删除学生信息的方法,使用了 JDBC 进行数据库操作。
具体来说,该方法接受两个参数:数据库连接对象 con 和要删除的学生信息的 id,使用占位符将 id 插入 SQL 语句中,然后通过 PreparedStatement 对象执行 SQL 语句,最后返回删除的行数。
需要注意的是,该方法一次可以删除多个学生信息,因为 SQL 语句中使用了 in 运算符,可以删除多个 id 对应的学生信息。
相关问题
解释代码//删除图书 public int bookDelete(Connection con, String id) throws Exception { String sql = "delete from t_book where id=?"; PreparedStatement pstmt = con.prepareStatement(sql); pstmt.setString(1, id); return pstmt.executeUpdate(); }
这段代码是一个在数据库中删除图书数据的方法,主要包括以下几个步骤:
1. 定义 SQL 语句:通过字符串拼接的方式定义 SQL 语句,其中使用了占位符 "?"。
2. 创建 PreparedStatement 对象:通过 Connection 对象的 prepareStatement 方法创建 PreparedStatement 对象,该对象可以执行 SQL 语句。
3. 设置参数:通过 PreparedStatement 对象的 setString 方法设置 SQL 语句中的占位符 "?" 的值,这里将图书的 id 作为参数传入。
4. 执行 SQL 语句:通过 PreparedStatement 对象的 executeUpdate 方法执行 SQL 语句,该方法返回被修改的记录行数。
5. 返回结果:将被修改的记录行数作为方法的返回值返回。
需要注意的是,在使用完 PreparedStatement 对象后需要手动关闭该对象以释放资源。
解释下列程序:public class collectDao { public int select(Connection con, int houseid)throws Exception{ int a = 0; String sql="select * from t_collect where houseId=? and cusid=? "; PreparedStatement pstmt=con.prepareStatement(sql); pstmt.setInt(1, houseid); pstmt.setInt(2, CustomerFrm.CUSID); ResultSet rs=pstmt.executeQuery(); if(rs.next()){ a = 1; } return a; } public int add(Connection con,collection c ) throws Exception{ String sql="insert into t_collect values(?,?)"; PreparedStatement pstmt=con.prepareStatement(sql); pstmt.setInt(1, c.getHouseId()); pstmt.setInt(2, c.getCusId()); return pstmt.executeUpdate(); } public int delete(Connection con,int houseId)throws Exception{ String sql="delete from t_collect where houseId=? and cusId=?"; PreparedStatement pstmt=con.prepareStatement(sql); pstmt.setInt(1, houseId); pstmt.setInt(2,CustomerFrm.CUSID); return pstmt.executeUpdate(); } }
这段程序是一个数据访问对象(DAO)类,用于操作名为“t_collect”的数据库表。它包含了三个方法:select、add和delete,分别对应了查询、添加和删除记录的操作。其中,select方法接受一个Connection对象和一个houseid参数,返回一个整数值(0或1),表示在t_collect表中是否存在满足houseId等于参数值houseid且cusid等于CustomerFrm.CUSID的记录。add方法接受一个Connection对象和一个collection对象,将collection对象中的houseId和cusId属性插入到t_collect表中。delete方法接受一个Connection对象和一个houseId参数,删除t_collect表中houseId等于参数值houseId且cusId等于CustomerFrm.CUSID的记录。在这些方法中,都使用了PreparedStatement对象执行SQL语句,并通过设置参数来避免SQL注入攻击。
相关推荐
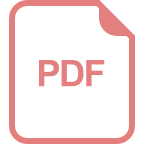
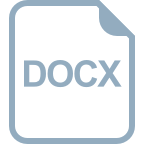
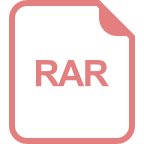













