stc32g12k128oled 屏幕代码怎么写
时间: 2024-02-01 11:13:25 浏览: 203
STC32G12K系列芯片并不直接支持OLED屏幕,因此需要使用外部驱动芯片来控制OLED屏幕。一般常用的OLED驱动芯片有SSD1306、SH1106等。这些驱动芯片都支持I2C或SPI接口传输数据。
以下是基于SSD1306驱动芯片的OLED屏幕代码示例:
```c
#include <stc12.h>
#define SCL P1_0
#define SDA P1_1
void i2c_delay() {
int i = 10;
while (i--);
}
void i2c_start() {
SDA = 1;
i2c_delay();
SCL = 1;
i2c_delay();
SDA = 0;
i2c_delay();
SCL = 0;
i2c_delay();
}
void i2c_stop() {
SDA = 0;
i2c_delay();
SCL = 1;
i2c_delay();
SDA = 1;
i2c_delay();
}
void i2c_write_byte(unsigned char dat) {
unsigned char i;
for (i = 0; i < 8; i++) {
if (dat & 0x80) {
SDA = 1;
}
else {
SDA = 0;
}
i2c_delay();
SCL = 1;
i2c_delay();
SCL = 0;
dat <<= 1;
}
SDA = 1;
i2c_delay();
SCL = 1;
i2c_delay();
SCL = 0;
}
void oled_init() {
i2c_start();
i2c_write_byte(0x78); //I2C地址,实际地址是0x3C,因为最低位是写入位
i2c_write_byte(0x00); //控制字节,表示后面要写入的是命令
i2c_write_byte(0xAE); //关闭显示
i2c_write_byte(0x20); //设置内存地址模式,00:水平模式,01:垂直模式,10:页模式
i2c_write_byte(0x10); //00:水平寻址模式,01:垂直寻址模式,10:页寻址模式
i2c_write_byte(0xb0); //设置显示起始行,0~7
i2c_write_byte(0xc8); //设置COM扫描方向,1:从上到下,0:从下到上
i2c_write_byte(0x00); //设置低列地址,由于我们使用的是128x64的屏幕,因此低列地址为0
i2c_write_byte(0x10); //设置高列地址,由于我们使用的是128x64的屏幕,因此高列地址为0
i2c_write_byte(0x40); //设置显示位置,行0~63
i2c_write_byte(0x81); //设置对比度,取值范围0~255
i2c_write_byte(0xff); //对比度值(0~255)
i2c_write_byte(0xa1); //设置段重定向,1:左右反转,0:正常显示
i2c_write_byte(0xa6); //设置显示方式,1:反白,0:正常显示
i2c_write_byte(0xa8); //设置多路复用率,1/64
i2c_write_byte(0x3f); //设置显示的页数,共64页
i2c_write_byte(0xa4); //关显示全局图
i2c_write_byte(0xd3); //设置显示偏移,0~63
i2c_write_byte(0x00); //不偏移
i2c_write_byte(0xd5); //设置显示时钟分频因子,震荡器频率,A[3:0]分别对应0,1,2,3
i2c_write_byte(0xf0); //分频因子和震荡器频率,低4位为分频因子,高4位为震荡器频率
i2c_write_byte(0xd9); //设置预充电期间,phase 1:1~15 phase 2:1~15
i2c_write_byte(0x22); //分别为phase 1和phase 2的调节周期,取值范围1~15,通常为2
i2c_write_byte(0xda); //设置COM硬件配置
i2c_write_byte(0x12); //设置COM硬件配置,bit[5]为1,表示扫描从COM63到COM0,bit[4]为1,表示启用COM左右反转,bit[0]为1,表示使能COM硬件配置
i2c_write_byte(0xdb); //设置VCOMH,可调节亮度
i2c_write_byte(0x40); //设置VCOMH,取值范围0x00~0x7f
i2c_write_byte(0x8d); //设置电源,控制电源器件,bit[0]为1,打开电源
i2c_write_byte(0x14); //通常设置为0x14
i2c_write_byte(0xaf); //开启显示
i2c_stop();
}
void oled_clear() {
unsigned char i, j;
for (i = 0; i < 8; i++) {
i2c_start();
i2c_write_byte(0x78); //I2C地址,实际地址是0x3C,因为最低位是写入位
i2c_write_byte(0x00); //控制字节,表示后面要写入的是命令
i2c_write_byte(0xb0 + i); //设置页地址
i2c_write_byte(0x00); //设置低列地址,列0~63
i2c_write_byte(0x10); //设置高列地址,列0~63
for (j = 0; j < 128; j++) {
i2c_write_byte(0x00); //写入0
}
i2c_stop();
}
}
void oled_show_string(unsigned char x, unsigned char y, unsigned char *str) {
unsigned char i = 0;
while (str[i] != '\0') {
oled_show_char(x + i * 6, y, str[i]);
i++;
}
}
void oled_show_char(unsigned char x, unsigned char y, unsigned char chr) {
unsigned char i = 0;
chr = chr - ' '; //计算字符在ASCII码表中的偏移量
i2c_start();
i2c_write_byte(0x78); //I2C地址,实际地址是0x3C,因为最低位是写入位
i2c_write_byte(0x00); //控制字节,表示后面要写入的是命令
i2c_write_byte(0xb0 + y); //设置页地址
i2c_write_byte((x & 0x0f) | 0x00); //设置低列地址,列0~63
i2c_write_byte(((x & 0xf0) >> 4) | 0x10); //设置高列地址,列0~63
for (i = 0; i < 6; i++) {
i2c_write_byte(font6x8[chr][i]);
}
i2c_stop();
}
void main() {
oled_init();
oled_clear();
oled_show_string(0, 0, "Hello, OLED!");
while (1);
}
```
需要注意的是,以上代码中的font6x8数组是一个6x8点阵字体数组,用于显示ASCII码表中的字符。如果需要显示其他字体或汉字,则需要使用相应的字模库。另外,以上代码中的I2C接口是使用软件模拟的方式实现的,如果需要使用硬件I2C接口,则需要根据具体的芯片型号和硬件接口进行相应的修改。
阅读全文
相关推荐
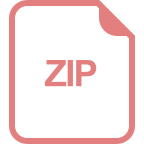
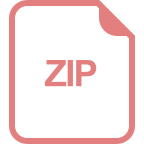



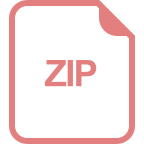
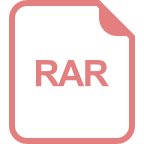
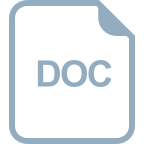
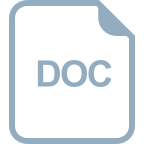


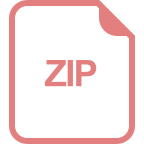
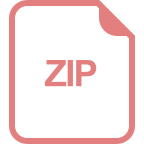
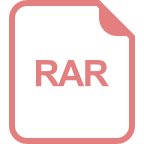
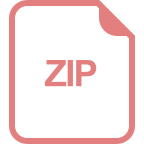
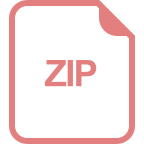