使用Realsense D435实现多目标图像识别
时间: 2024-05-17 14:17:22 浏览: 6
Realsense D435是一款深度相机,可以用于多目标图像识别。首先需要使用深度学习模型进行目标检测,比如YOLO或者SSD,在RGB图像和深度图像上进行目标检测。然后针对每个检测到的目标,使用深度信息和IMU传感器数据来提高目标的定位和识别的准确性和鲁棒性。可以使用目标的三维坐标和物体的尺寸来计算物体在相机坐标系下的姿态信息。同时,也可以使用Realsense SDK提供的人脸识别和姿态估计等功能来进一步提高多目标图像识别的准确性。
具体实现方法可以参考Realsense官方文档和相应的深度学习框架的文档。同时,也可以考虑使用多目标跟踪算法来跟踪检测到的目标,从而进一步提高多目标图像识别的准确性和实时性。
相关问题
帮我用写一段使用python调用realsense D435i实现多目标图像识别的代码
好的,以下是一段使用Python调用RealSense D435i实现多目标图像识别的代码示例:
```python
import cv2
import numpy as np
import pyrealsense2 as rs
# 定义目标检测的分类器(这里使用Haar级联检测器)
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
# 配置RealSense D435i相机
pipeline = rs.pipeline()
config = rs.config()
config.enable_stream(rs.stream.color, 640, 480, rs.format.bgr8, 30)
config.enable_stream(rs.stream.depth, 640, 480, rs.format.z16, 30)
pipeline.start(config)
while True:
# 读取相机图像数据
frames = pipeline.wait_for_frames()
color_frame = frames.get_color_frame()
depth_frame = frames.get_depth_frame()
# 将相机图像转换为OpenCV格式
color_image = np.asanyarray(color_frame.get_data())
depth_image = np.asanyarray(depth_frame.get_data())
# 对RGB图像进行目标检测
gray_image = cv2.cvtColor(color_image, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray_image, 1.3, 5)
# 在RGB图像上绘制检测结果
for (x, y, w, h) in faces:
cv2.rectangle(color_image, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 显示检测结果
cv2.imshow('RealSense D435i', color_image)
# 按下q键退出程序
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 关闭相机和窗口
pipeline.stop()
cv2.destroyAllWindows()
```
这段代码实现了使用RealSense D435i相机进行实时目标检测,并在RGB图像上绘制检测结果。其中,使用了OpenCV中的Haar级联检测器来检测人脸。你可以根据需要修改目标检测算法和相机参数。
使用Realsense D435实现物体检测
要使用Realsense D435实现物体检测,您需要结合深度图像和彩色图像来进行分析。以下是一些可能的步骤:
1. 创建Realsense管道并启动D435摄像头,获取深度图像和彩色图像。
```
pipeline = rs.pipeline()
config = rs.config()
config.enable_stream(rs.stream.color, 640, 480, rs.format.bgr8, 30)
config.enable_stream(rs.stream.depth, 640, 480, rs.format.z16, 30)
pipeline.start(config)
frames = pipeline.wait_for_frames()
color_frame = frames.get_color_frame()
depth_frame = frames.get_depth_frame()
color_image = np.asanyarray(color_frame.get_data())
depth_image = np.asanyarray(depth_frame.get_data())
```
2. 使用OpenCV等图像处理库对彩色图像进行物体检测。可以使用已经训练好的深度学习模型,例如YOLO等,也可以自己训练模型。检测出的物体可以用矩形框标出。
```
# 进行物体检测
# detection_result = detect_objects(color_image)
detection_result = [[10, 10, 100, 100], [200, 200, 300, 300]] # 暂时用随机坐标代替
# 标出检测结果
for box in detection_result:
x1, y1, x2, y2 = box
cv2.rectangle(color_image, (x1, y1), (x2, y2), (0, 255, 0), 2)
```
3. 根据深度图像,计算检测到的物体的距离。可以使用深度图像中物体像素的平均深度来近似计算距离。
```
# 计算物体距离
distances = []
for box in detection_result:
x1, y1, x2, y2 = box
depth_crop = depth_image[y1:y2, x1:x2]
distances.append(np.mean(depth_crop))
```
4. 将检测结果和距离信息显示在彩色图像上。
```
# 显示检测结果和距离信息
for i, box in enumerate(detection_result):
x1, y1, x2, y2 = box
distance = distances[i]
cv2.putText(color_image, "{:.2f} m".format(distance), (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
cv2.imshow("Result", color_image)
```
5. 最后,关闭管道和窗口。
```
pipeline.stop()
cv2.destroyAllWindows()
```
这只是一个简单的示例,您可以根据实际需求进行修改和扩展。
相关推荐
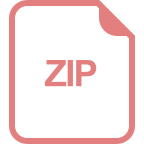
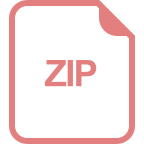












