使用realsense d435、opencv和python写一个简单的数字识别代码
时间: 2024-04-08 11:33:11 浏览: 14
当然可以!以下是一个简单的数字识别代码示例,使用Realsense D435相机、OpenCV和Python:
```python
import cv2
import numpy as np
# 加载数字分类器
classifier = cv2.ml.KNearest_create()
classifier.load('digits_classifier.xml')
# 初始化Realsense相机
pipeline = cv2.rs.pipeline()
config = cv2.rs.config()
config.enable_stream(cv2.rs.stream.color, 640, 480, cv2.rs.format.bgr8, 30)
pipeline.start(config)
while True:
# 等待获取图像
frames = pipeline.wait_for_frames()
color_frame = frames.get_color_frame()
if not color_frame:
continue
# 将图像转为OpenCV格式
frame = np.asanyarray(color_frame.get_data())
# 转为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 进行阈值处理,以便提取数字
_, threshold = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 查找数字轮廓
contours, _ = cv2.findContours(threshold.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
# 获取数字的边界框
(x, y, w, h) = cv2.boundingRect(contour)
# 提取数字区域
digit = threshold[y:y+h, x:x+w]
# 调整数字大小
resized_digit = cv2.resize(digit, (28, 28))
# 展平图像为一维向量
flatten_digit = resized_digit.reshape((1, 28 * 28))
# 转换为浮点型并进行归一化
flatten_digit = np.float32(flatten_digit) / 255.0
# 使用分类器进行数字识别
_, result, _, _ = classifier.findNearest(flatten_digit, k=1)
# 获取识别结果
digit_label = int(result[0][0])
# 绘制识别结果
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
cv2.putText(frame, str(digit_label), (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
# 显示图像
cv2.imshow("Digit Recognition", frame)
# 按下ESC键退出
if cv2.waitKey(1) == 27:
break
# 关闭相机和窗口
pipeline.stop()
cv2.destroyAllWindows()
```
请确保已经训练了一个数字分类器,并将其保存为`digits_classifier.xml`文件。以上代码将实时从Realsense D435相机获取图像,并使用数字分类器识别图像中的数字。识别结果将在图像上进行绘制。
注意:这只是一个简单的示例,你可能需要根据你的实际需求进行修改和优化。同时确保已经正确安装了Realsense SDK和OpenCV库。
相关推荐
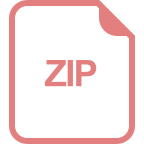
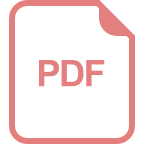














