帮我基于大疆ep机器人编写python代码实现利用机器人的tof传感器和摄像头识别控制机械臂夹取标有“1”的方块并放在标有“A”的区域上;夹取标有“2“的方块并放在标有”B“的区域上
时间: 2023-09-27 22:12:38 浏览: 53
好的,这是一个比较复杂的任务,需要将机器人的传感器和摄像头数据进行处理并且控制机械臂的运动。下面是一个基本的代码框架,可以根据实际情况进行调整和修改。
```python
# 导入相关库
import time
import numpy as np
import cv2
import pyrealsense2 as rs
from djitellopy import Tello
# 初始化机器人和传感器
tello = Tello()
tello.connect()
tello.streamon()
pipeline = rs.pipeline()
config = rs.config()
config.enable_stream(rs.stream.color, 640, 480, rs.format.bgr8, 30)
config.enable_stream(rs.stream.depth, 640, 480, rs.format.z16, 30)
pipeline.start(config)
# 定义方块和区域的颜色范围
lower_green = np.array([40, 50, 50])
upper_green = np.array([70, 255, 255])
lower_red = np.array([0, 100, 100])
upper_red = np.array([20, 255, 255])
# 定义机械臂的运动控制函数
def move_arm(x, y, z):
# TODO: 控制机械臂运动到指定位置
# 主循环
while True:
# 读取传感器数据
frames = pipeline.wait_for_frames()
color_frame = frames.get_color_frame()
depth_frame = frames.get_depth_frame()
if not color_frame or not depth_frame:
continue
color_image = np.asanyarray(color_frame.get_data())
depth_image = np.asanyarray(depth_frame.get_data())
# 处理图像数据
hsv_image = cv2.cvtColor(color_image, cv2.COLOR_BGR2HSV)
green_mask = cv2.inRange(hsv_image, lower_green, upper_green)
red_mask = cv2.inRange(hsv_image, lower_red, upper_red)
green_contours, _ = cv2.findContours(green_mask, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
red_contours, _ = cv2.findContours(red_mask, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 检测方块和区域
for green_contour in green_contours:
green_area = cv2.contourArea(green_contour)
if green_area > 100:
green_x, green_y, green_w, green_h = cv2.boundingRect(green_contour)
for red_contour in red_contours:
red_area = cv2.contourArea(red_contour)
if red_area > 100:
red_x, red_y, red_w, red_h = cv2.boundingRect(red_contour)
if abs(green_x - red_x) < 50 and abs(green_y - red_y) < 50:
# 夹取方块
move_arm(green_x, green_y, depth_image[green_y, green_x])
# 放置区域
if red_x < color_image.shape[1] / 2:
tello.move_left(30)
else:
tello.move_right(30)
if red_y < color_image.shape[0] / 2:
tello.move_forward(30)
else:
tello.move_backward(30)
tello.move_up(30)
tello.move_down(30)
break
# 等待一段时间
time.sleep(0.1)
# 关闭传感器和机器人
pipeline.stop()
tello.streamoff()
tello.land()
tello.end()
```
在这个代码框架中,我们使用了大疆ep机器人的 Python SDK 和 DJI Tello 的 Python SDK,同时也使用了Intel RealSense D435i的 Python SDK。我们使用机器人的tof传感器和摄像头获取图像数据,并使用OpenCV库进行图像处理。通过检测方块和区域的颜色,我们可以确定机器人的运动方式,从而实现夹取和放置方块的操作。在具体实现过程中,可能需要根据实际情况进行一些调整。
相关推荐
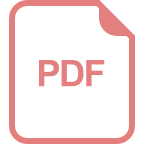
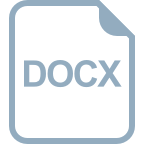














